springboot fastjson 设置时区
时间: 2023-10-19 15:34:49 浏览: 143
在 Spring Boot 中,可以通过配置文件或代码设置 Fastjson 的时区。以下是两种常见的设置方法:
1. 通过配置文件设置时区:
在 `application.properties` 或 `application.yml` 中添加以下配置:
```properties
spring.fastjson.date-format=yyyy-MM-dd HH:mm:ss
spring.fastjson.time-zone=GMT+8
```
通过上述配置,可以设置 Fastjson 的默认日期格式为 "yyyy-MM-dd HH:mm:ss",时区为 GMT+8。
2. 通过代码设置时区:
在启动类中,可以通过调用 `JSON.DEFFAULT_TIME_ZONE` 方法来设置 Fastjson 的时区:
```java
import com.alibaba.fastjson.JSON;
public class YourApplication {
public static void main(String[] args) {
JSON.DEFAULT_TIME_ZONE = "GMT+8";
SpringApplication.run(YourApplication.class, args);
}
}
```
在上述代码中,将 Fastjson 的默认时区设置为 GMT+8。
请根据你的实际需求选择其中一种方法进行时区的设置。
相关推荐
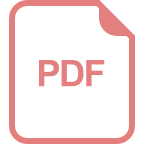
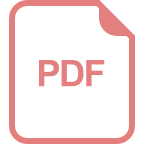
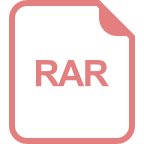
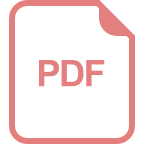
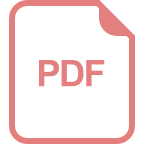
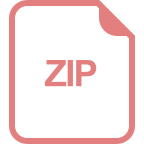
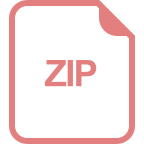
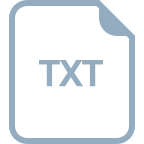
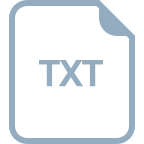
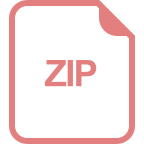
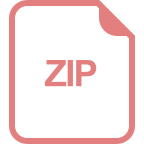
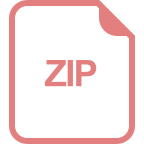
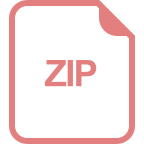