怎样输出yolov5检测的fps
时间: 2023-07-12 16:13:18 浏览: 172
要输出Yolov5检测的FPS,你可以使用以下代码:
```python
import time
import cv2
import torch
from models.experimental import attempt_load
from utils.general import non_max_suppression
# Load YOLOv5 model
weights = 'yolov5s.pt'
device = 'cuda' if torch.cuda.is_available() else 'cpu'
model = attempt_load(weights, map_location=device)
stride = int(model.stride.max()) # model stride
names = model.module.names if hasattr(model, 'module') else model.names # get class names
# Open video file
cap = cv2.VideoCapture('video.mp4')
# Loop through frames
start = time.time()
while True:
ret, frame = cap.read()
if not ret:
break
# Format image
img = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
img = cv2.resize(img, (640, 640))
img = img.transpose(2, 0, 1)
img = torch.from_numpy(img).float().div(255.0).unsqueeze(0)
# Run inference
pred = model(img, augment=False)[0]
pred = non_max_suppression(pred, conf_thres=0.25, iou_thres=0.45, classes=None, agnostic=False)
# Draw predictions
for i, det in enumerate(pred):
if det is not None and len(det):
# Rescale boxes from img_size to frame size
det[:, :4] = det[:, :4] * stride
box_colors = [[0, 255, 0], [0, 0, 255], [255, 0, 0]]
for *xyxy, conf, cls in reversed(det):
# Add bbox to the frame
label = f'{names[int(cls)]} {conf:.2f}'
color = box_colors[int(cls) % 3]
line_thickness = 2
cv2.rectangle(frame, [int(x) for x in xyxy], color, thickness=line_thickness)
cv2.putText(frame, label, (int(xyxy[0]), int(xyxy[1] - 10)), cv2.FONT_HERSHEY_SIMPLEX, 0.5, color, line_thickness)
# Display frame
cv2.imshow('frame', frame)
if cv2.waitKey(1) == ord('q'):
break
# Release resources
cap.release()
cv2.destroyAllWindows()
# Calculate FPS
end = time.time()
print(f'FPS: {int(cap.get(cv2.CAP_PROP_FRAME_COUNT) / (end - start))}')
```
这段代码使用OpenCV读取视频文件并循环遍历每一帧。对于每一帧,它将图像格式化,运行YOLOv5模型进行推理,然后使用OpenCV在每个检测到的物体周围绘制边界框和标签。最后,它显示帧并计算并输出FPS。
阅读全文
相关推荐
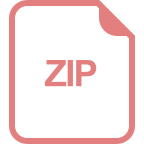
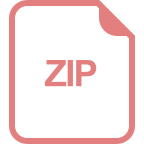
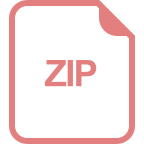


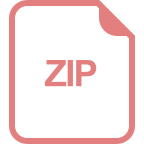






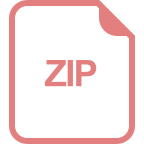




