java 使用 itext调用打印机打印 pdf
时间: 2023-08-31 08:21:58 浏览: 140
### 回答1:
使用iText打印PDF文件可以通过以下步骤实现:
1. 导入iText库
首先,需要将iText库添加到Java项目中。可以在Maven中添加以下依赖项:
```
<dependency>
<groupId>com.itextpdf</groupId>
<artifactId>itextpdf</artifactId>
<version>5.5.13</version>
</dependency>
```
或者可以从iText的官方网站下载库并手动添加到项目中。
2. 创建PDF文档
使用iText创建PDF文档。这可以通过以下代码实现:
```
Document document = new Document();
PdfWriter writer = PdfWriter.getInstance(document, new FileOutputStream("sample.pdf"));
document.open();
document.add(new Paragraph("Hello World!"));
document.close();
writer.close();
```
这段代码创建一个包含“Hello World!”文本的PDF文档。
3. 打印PDF文件
使用Java的PrintService类来获取系统中的打印机,并使用PdfReader类读取创建的PDF文档。以下代码演示了如何实现:
```
PrintService[] printers = PrintServiceLookup.lookupPrintServices(null, null);
PdfReader reader = new PdfReader("sample.pdf");
PrintService printer = printers[0]; // 选择系统中的第一个打印机
DocPrintJob job = printer.createPrintJob();
PdfPrint pdfPrint = new PdfPrint(reader, job);
pdfPrint.print();
```
这段代码选择系统中的第一个打印机,并使用PdfPrint类来打印PDF文档。注意,在运行此代码之前,确保有打印机可用。
以上是使用iText在Java中调用打印机打印PDF文件的基本步骤。您可以根据自己的需求对其进行修改和调整。
### 回答2:
使用iText调用打印机打印PDF,可以通过以下步骤实现:
1. 首先,确保已经正确安装和配置了iText库,并将其添加到Java项目中的类路径中。
2. 创建一个空白的PDF文档对象,可以使用`PdfWriter`类来实现,通过指定输出流将其与文件关联起来。
3. 使用iText的API来添加文本、图片、表格等内容到PDF文档中。这些内容可以使用`com.itextpdf.text`包中的相应类来创建和设置。
4. 在将PDF文档打印之前,需要首先将其导出为临时文件。可以使用`java.io.File`类来生成一个带有唯一名称的临时文件,并将PDF内容写入该文件。
5. 调用系统的打印机服务,使用Java的`java.awt.Desktop.print(File file)`方法,将PDF文件发送给打印机进行打印。
下面是一个示例代码,演示了如何使用iText将PDF文档打印到打印机:
```java
import com.itextpdf.text.Document;
import com.itextpdf.text.Paragraph;
import com.itextpdf.text.pdf.PdfWriter;
import java.awt.*;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
public class PrintPdfWithIText {
public static void main(String[] args) {
// 创建PDF文档
Document document = new Document();
try {
// 绑定到一个临时PDF文件
File outputFile = File.createTempFile("temp", ".pdf");
PdfWriter.getInstance(document, new FileOutputStream(outputFile));
// 打开文档
document.open();
// 添加内容
document.add(new Paragraph("Hello, World!"));
// 关闭文档
document.close();
// 打印PDF文件
if (Desktop.isDesktopSupported()) {
Desktop.getDesktop().print(outputFile);
}
} catch (IOException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
这是一个简单的示例代码,演示了使用iText创建一个包含"Hello, World!"文本的PDF文档,并将其打印到默认打印机。可以根据具体需求修改和扩展代码。
### 回答3:
Java可以使用iText库来调用打印机打印PDF文件。iText是一个功能强大的Java库,可以用于创建、操纵和处理PDF文档。
使用iText调用打印机打印PDF文件的步骤如下:
1. 首先,需要通过下载iText库的jar文件并将其导入到Java项目中。
2. 创建一个打印任务,并指定要打印的PDF文件的路径。可以使用FileInputStream类来读取PDF文件。
3. 创建一个打印机的选择对话框,以显示可用的打印机列表供用户选择。可以使用javax.print包中的类来实现该功能。
4. 获取用户选择的打印机,并将其作为参数传递给PrintServiceLookup类的lookupDefaultPrintService方法。
5. 创建一个DocFlavor对象,指定打印的文档类型为PDF。可以使用javax.print包中的DocFlavor类来实现。
6. 创建一个Doc对象,将读取的PDF文件和DocFlavor对象作为参数传递给Doc类的构造函数。
7. 创建一个PrintJob对象,并将打印机和Doc对象作为参数传递给PrintService类的createPrintJob方法。
8. 调用PrintJob对象的print方法,开始进行打印操作。
9. 最后,关闭文件输入流和打印作业。
使用iText库调用打印机打印PDF文件可以实现灵活的打印功能,可以选择不同的打印机和页面设置,同时也能够处理PDF文档的内容和样式。通过iText,Java可以轻松地实现PDF文件的打印操作。
相关推荐
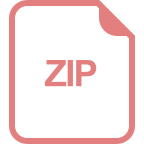
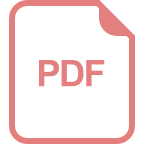












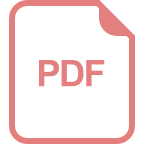