根据Service及BroadcastReceiver的知识讲解和案例使用,实现一个Android音乐播放器,要求能实现音乐的播放,暂停,上一首,下一首等功能。使用Kotlin,音乐数据源为raw文件夹下的
时间: 2023-11-28 13:04:25 浏览: 121
首先,我们需要在项目的 `res` 目录下创建一个 `raw` 文件夹,并将音乐文件放入其中。
接下来,我们需要创建一个 `MusicService` 类来实现音乐的播放、暂停、上一首和下一首等功能。这个类需要继承自 `Service` 类,重写 `onBind()` 和 `onStartCommand()` 方法。
在 `onBind()` 方法中,我们需要返回一个 `null` 值,因为我们不需要在 `Activity` 中绑定这个服务。
在 `onStartCommand()` 方法中,我们需要根据传入的指令进行相应的操作。我们可以定义一些常量来表示各种指令,比如 `ACTION_PLAY`、`ACTION_PAUSE`、`ACTION_PREVIOUS` 和 `ACTION_NEXT`。当接收到这些指令时,我们可以调用相应的方法来实现音乐的播放、暂停、上一首和下一首等功能。
在 `MusicService` 类中,我们还需要定义一个 `MediaPlayer` 对象来播放音乐。在播放音乐时,我们需要先释放之前的 `MediaPlayer` 对象,然后重新创建一个新的 `MediaPlayer` 对象并开始播放音乐。
接下来,我们需要创建一个 `MusicBroadcastReceiver` 类来接收来自 `Activity` 的指令,并将指令发送给 `MusicService`。这个类需要继承自 `BroadcastReceiver` 类,重写 `onReceive()` 方法。
在 `onReceive()` 方法中,我们需要获取传递过来的指令,并使用 `Intent` 将指令发送给 `MusicService`。我们可以使用 `startService()` 方法来启动 `MusicService` 并传递指令。
最后,我们需要在 `Activity` 中创建一个布局文件来显示音乐播放器的界面,并使用 `IntentFilter` 和 `registerReceiver()` 方法来注册 `MusicBroadcastReceiver`。
以下是具体的实现代码:
MusicService.kt
```kotlin
class MusicService : Service() {
companion object {
const val ACTION_PLAY = "com.example.musicplayer.action.PLAY"
const val ACTION_PAUSE = "com.example.musicplayer.action.PAUSE"
const val ACTION_PREVIOUS = "com.example.musicplayer.action.PREVIOUS"
const val ACTION_NEXT = "com.example.musicplayer.action.NEXT"
}
private var mediaPlayer: MediaPlayer? = null
private var currentSongId = -1
private lateinit var songs: IntArray
override fun onBind(intent: Intent?): IBinder? {
return null
}
override fun onStartCommand(intent: Intent?, flags: Int, startId: Int): Int {
intent?.let {
when (it.action) {
ACTION_PLAY -> {
val songId = it.getIntExtra("songId", -1)
if (songId != currentSongId) {
currentSongId = songId
mediaPlayer?.release()
mediaPlayer = MediaPlayer.create(this, songs[songId])
mediaPlayer?.start()
} else {
mediaPlayer?.start()
}
}
ACTION_PAUSE -> {
mediaPlayer?.pause()
}
ACTION_PREVIOUS -> {
currentSongId = (currentSongId - 1 + songs.size) % songs.size
mediaPlayer?.release()
mediaPlayer = MediaPlayer.create(this, songs[currentSongId])
mediaPlayer?.start()
}
ACTION_NEXT -> {
currentSongId = (currentSongId + 1) % songs.size
mediaPlayer?.release()
mediaPlayer = MediaPlayer.create(this, songs[currentSongId])
mediaPlayer?.start()
}
}
}
return START_NOT_STICKY
}
override fun onDestroy() {
mediaPlayer?.release()
super.onDestroy()
}
override fun onTaskRemoved(rootIntent: Intent?) {
mediaPlayer?.release()
stopSelf()
super.onTaskRemoved(rootIntent)
}
override fun onCreate() {
super.onCreate()
songs = intArrayOf(R.raw.song1, R.raw.song2, R.raw.song3, R.raw.song4)
}
}
```
MusicBroadcastReceiver.kt
```kotlin
class MusicBroadcastReceiver : BroadcastReceiver() {
override fun onReceive(context: Context, intent: Intent) {
val action = intent.action ?: return
val serviceIntent = Intent(context, MusicService::class.java)
when (action) {
MusicService.ACTION_PLAY -> {
val songId = intent.getIntExtra("songId", -1)
if (songId != -1) {
serviceIntent.putExtra("songId", songId)
}
}
MusicService.ACTION_PAUSE -> {
}
MusicService.ACTION_PREVIOUS -> {
}
MusicService.ACTION_NEXT -> {
}
}
serviceIntent.action = action
ContextCompat.startForegroundService(context, serviceIntent)
}
}
```
MusicPlayerActivity.kt
```kotlin
class MusicPlayerActivity : AppCompatActivity() {
private lateinit var broadcastReceiver: MusicBroadcastReceiver
private lateinit var playButton: ImageButton
private lateinit var pauseButton: ImageButton
private lateinit var previousButton: ImageButton
private lateinit var nextButton: ImageButton
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_music_player)
playButton = findViewById(R.id.play_button)
pauseButton = findViewById(R.id.pause_button)
previousButton = findViewById(R.id.previous_button)
nextButton = findViewById(R.id.next_button)
broadcastReceiver = MusicBroadcastReceiver()
val intentFilter = IntentFilter().apply {
addAction(MusicService.ACTION_PLAY)
addAction(MusicService.ACTION_PAUSE)
addAction(MusicService.ACTION_PREVIOUS)
addAction(MusicService.ACTION_NEXT)
}
registerReceiver(broadcastReceiver, intentFilter)
playButton.setOnClickListener {
val intent = Intent(MusicService.ACTION_PLAY).apply {
putExtra("songId", 0)
}
sendBroadcast(intent)
}
pauseButton.setOnClickListener {
val intent = Intent(MusicService.ACTION_PAUSE)
sendBroadcast(intent)
}
previousButton.setOnClickListener {
val intent = Intent(MusicService.ACTION_PREVIOUS)
sendBroadcast(intent)
}
nextButton.setOnClickListener {
val intent = Intent(MusicService.ACTION_NEXT)
sendBroadcast(intent)
}
}
override fun onDestroy() {
unregisterReceiver(broadcastReceiver)
super.onDestroy()
}
}
```
阅读全文
相关推荐
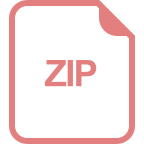
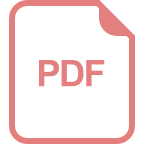
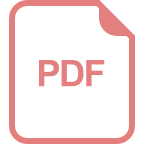















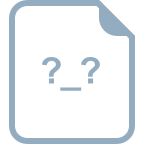