用swing写一个超市管理系统的增删改查
时间: 2023-10-06 20:06:49 浏览: 82
好的,下面是一个简单的超市管理系统的增删改查的Java Swing实现:
```java
import java.awt.EventQueue;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
import java.util.List;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.JTextField;
import javax.swing.table.DefaultTableModel;
public class SupermarketManagementSystem {
private JFrame frame;
private JTable table;
private JTextField textFieldName;
private JTextField textFieldPrice;
private JTextField textFieldQuantity;
private List<Product> products = new ArrayList<Product>();
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
SupermarketManagementSystem window = new SupermarketManagementSystem();
window.frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the application.
*/
public SupermarketManagementSystem() {
initialize();
}
/**
* Initialize the contents of the frame.
*/
private void initialize() {
frame = new JFrame();
frame.setBounds(100, 100, 600, 400);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.getContentPane().setLayout(null);
JLabel lblNewLabel = new JLabel("Name:");
lblNewLabel.setBounds(10, 10, 50, 20);
frame.getContentPane().add(lblNewLabel);
textFieldName = new JTextField();
textFieldName.setBounds(70, 10, 100, 20);
frame.getContentPane().add(textFieldName);
textFieldName.setColumns(10);
JLabel lblPrice = new JLabel("Price:");
lblPrice.setBounds(10, 40, 50, 20);
frame.getContentPane().add(lblPrice);
textFieldPrice = new JTextField();
textFieldPrice.setBounds(70, 40, 100, 20);
frame.getContentPane().add(textFieldPrice);
textFieldPrice.setColumns(10);
JLabel lblQuantity = new JLabel("Quantity:");
lblQuantity.setBounds(10, 70, 50, 20);
frame.getContentPane().add(lblQuantity);
textFieldQuantity = new JTextField();
textFieldQuantity.setBounds(70, 70, 100, 20);
frame.getContentPane().add(textFieldQuantity);
textFieldQuantity.setColumns(10);
JButton btnAdd = new JButton("Add");
btnAdd.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String name = textFieldName.getText();
double price = Double.parseDouble(textFieldPrice.getText());
int quantity = Integer.parseInt(textFieldQuantity.getText());
Product product = new Product(name, price, quantity);
products.add(product);
updateTable();
}
});
btnAdd.setBounds(10, 100, 80, 20);
frame.getContentPane().add(btnAdd);
JButton btnDelete = new JButton("Delete");
btnDelete.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
int selectedRow = table.getSelectedRow();
if (selectedRow >= 0) {
products.remove(selectedRow);
updateTable();
}
}
});
btnDelete.setBounds(100, 100, 80, 20);
frame.getContentPane().add(btnDelete);
JButton btnUpdate = new JButton("Update");
btnUpdate.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
int selectedRow = table.getSelectedRow();
if (selectedRow >= 0) {
String name = textFieldName.getText();
double price = Double.parseDouble(textFieldPrice.getText());
int quantity = Integer.parseInt(textFieldQuantity.getText());
Product product = new Product(name, price, quantity);
products.set(selectedRow, product);
updateTable();
}
}
});
btnUpdate.setBounds(190, 100, 80, 20);
frame.getContentPane().add(btnUpdate);
JScrollPane scrollPane = new JScrollPane();
scrollPane.setBounds(10, 130, 560, 220);
frame.getContentPane().add(scrollPane);
table = new JTable();
table.setModel(new DefaultTableModel(new Object[][] {}, new String[] { "Name", "Price", "Quantity" }) {
private static final long serialVersionUID = 1L;
Class<?>[] columnTypes = new Class[] { String.class, Double.class, Integer.class };
public Class<?> getColumnClass(int columnIndex) {
return columnTypes[columnIndex];
}
boolean[] columnEditables = new boolean[] { false, false, false };
public boolean isCellEditable(int row, int column) {
return columnEditables[column];
}
});
scrollPane.setViewportView(table);
}
private void updateTable() {
DefaultTableModel model = (DefaultTableModel) table.getModel();
model.setRowCount(0);
for (Product product : products) {
model.addRow(new Object[] { product.getName(), product.getPrice(), product.getQuantity() });
}
}
private static class Product {
private String name;
private double price;
private int quantity;
public Product(String name, double price, int quantity) {
this.name = name;
this.price = price;
this.quantity = quantity;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public int getQuantity() {
return quantity;
}
public void setQuantity(int quantity) {
this.quantity = quantity;
}
}
}
```
这个示例中,我们创建了一个 `Product` 类来表示超市的商品,然后使用 `List` 来存储所有的商品。在 `initialize()` 方法中,我们创建了一个包含 `JLabel` 、`JTextField` 、`JButton` 和 `JTable` 的 `JFrame`。我们使用 `JTextField` 来输入商品的名称、价格和数量,使用 `JButton` 来添加、删除和更新商品,使用 `JTable` 来显示所有商品的信息。我们使用 `DefaultTableModel` 来管理 `JTable` 中的数据,并使用 `updateTable()` 方法来更新 `JTable` 中的数据。当用户点击添加、删除或更新按钮时,我们将读取 `JTextField` 中的数据,创建一个新的 `Product` 对象,并将其添加到 `List` 中或更新 `List` 中的现有对象。最后,我们调用 `updateTable()` 方法来更新 `JTable` 中的数据。
阅读全文
相关推荐
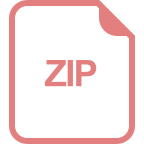
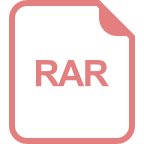
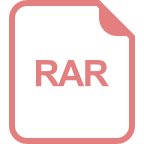
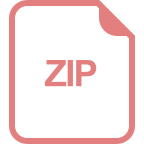
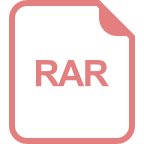
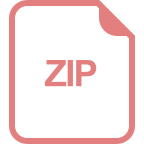
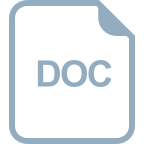
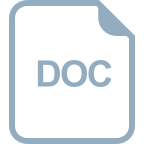
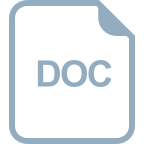
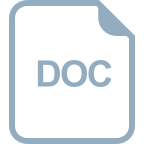
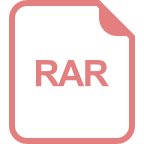
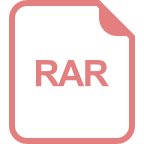
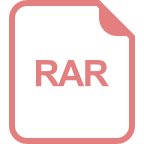
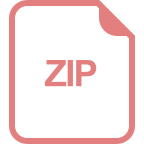
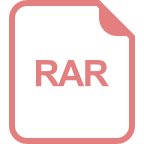