现有一个List<BarCodeRedisDTO> dtos ,BarCodeRedisDTO中有goodsCode、goodsPrice。现在需要统计出每一个goodsCode有几个。返回一个OrderUserCashBackGoodsInfoDto,OrderUserCashBackGoodsInfoDto中有goodsCode、goodsPrice、count。count就表示同一个goodsCode的数量
时间: 2024-03-10 19:45:00 浏览: 62
好的,根据你的要求,我可以给出以下代码实现:
```java
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class Main {
public static void main(String[] args) {
// 假设这是你的List<BarCodeRedisDTO> dtos
List<BarCodeRedisDTO> dtos = new ArrayList<>();
dtos.add(new BarCodeRedisDTO("code1", 10));
dtos.add(new BarCodeRedisDTO("code2", 20));
dtos.add(new BarCodeRedisDTO("code1", 10));
dtos.add(new BarCodeRedisDTO("code3", 30));
dtos.add(new BarCodeRedisDTO("code1", 10));
// 统计每一个goodsCode的数量
Map<String, Integer> countMap = new HashMap<>();
for (BarCodeRedisDTO dto : dtos) {
String goodsCode = dto.getGoodsCode();
int count = countMap.getOrDefault(goodsCode, 0);
countMap.put(goodsCode, count + 1);
}
// 构造返回结果
List<OrderUserCashBackGoodsInfoDto> result = new ArrayList<>();
for (BarCodeRedisDTO dto : dtos) {
String goodsCode = dto.getGoodsCode();
int goodsPrice = dto.getGoodsPrice();
int count = countMap.get(goodsCode);
OrderUserCashBackGoodsInfoDto infoDto = new OrderUserCashBackGoodsInfoDto(goodsCode, goodsPrice, count);
// 防止重复添加
if (!result.contains(infoDto)) {
result.add(infoDto);
}
}
// 打印结果
for (OrderUserCashBackGoodsInfoDto infoDto : result) {
System.out.println(infoDto);
}
}
}
class BarCodeRedisDTO {
private String goodsCode;
private int goodsPrice;
public BarCodeRedisDTO(String goodsCode, int goodsPrice) {
this.goodsCode = goodsCode;
this.goodsPrice = goodsPrice;
}
public String getGoodsCode() {
return goodsCode;
}
public int getGoodsPrice() {
return goodsPrice;
}
}
class OrderUserCashBackGoodsInfoDto {
private String goodsCode;
private int goodsPrice;
private int count;
public OrderUserCashBackGoodsInfoDto(String goodsCode, int goodsPrice, int count) {
this.goodsCode = goodsCode;
this.goodsPrice = goodsPrice;
this.count = count;
}
public String getGoodsCode() {
return goodsCode;
}
public int getGoodsPrice() {
return goodsPrice;
}
public int getCount() {
return count;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null || getClass() != obj.getClass()) {
return false;
}
OrderUserCashBackGoodsInfoDto that = (OrderUserCashBackGoodsInfoDto) obj;
return goodsCode.equals(that.goodsCode);
}
@Override
public int hashCode() {
return goodsCode.hashCode();
}
@Override
public String toString() {
return "OrderUserCashBackGoodsInfoDto{" +
"goodsCode='" + goodsCode + '\'' +
", goodsPrice=" + goodsPrice +
", count=" + count +
'}';
}
}
```
代码中使用了Map来统计每一个goodsCode的数量,然后遍历原List构造返回结果,并防止重复添加。最后打印结果。由于这里只是一个示例,你需要根据实际情况进行修改。
阅读全文
相关推荐
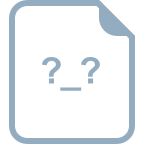
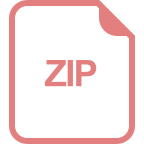
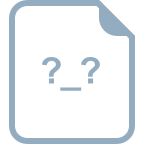


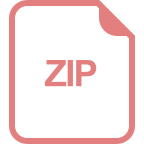
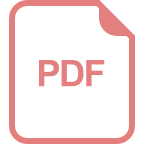










