DSR协议实现代码 python
时间: 2023-07-23 16:03:33 浏览: 118
DSR协议的实现需要用到路由表、缓存表、发送和接收数据包等。下面是一个简单的 Python 实现:
```python
# 路由表
route_table = {}
# 缓存表
cache_table = {}
# 发送数据包
def send_packet(source, destination, data):
route = find_route(source, destination)
if route:
next_hop = route[0]
packet = {
'source': source,
'destination': destination,
'data': data
}
send_to_next_hop(next_hop, packet)
else:
print('No route found for', destination)
# 查找路由
def find_route(source, destination):
if destination in route_table:
return route_table[destination]
elif source in cache_table:
return cache_table[source]
else:
return None
# 发送到下一个节点
def send_to_next_hop(next_hop, packet):
# 省略发送逻辑
pass
# 接收数据包
def receive_packet(node, packet):
if packet['destination'] == node:
process_data(packet['source'], packet['data'])
else:
cache_packet(node, packet)
# 处理数据
def process_data(source, data):
# 省略处理逻辑
pass
# 缓存数据包
def cache_packet(node, packet):
if node not in cache_table:
cache_table[node] = []
cache_table[node].append(packet)
```
这只是一个基本的实现,实际上 DSR 协议还有许多细节需要考虑,例如路由发现、路由维护等。
阅读全文
相关推荐
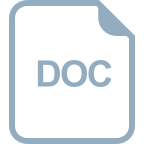
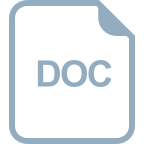
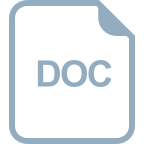




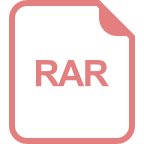
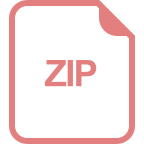
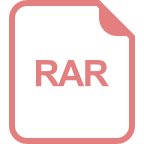
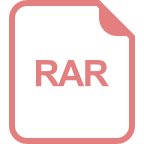
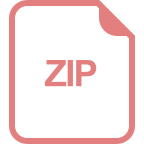
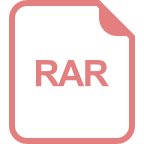
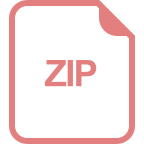
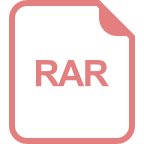
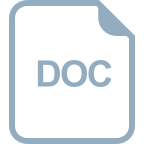
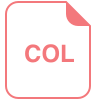
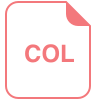
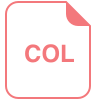