用jsp+sql写一个简单的图书管理系统
时间: 2024-03-06 12:50:25 浏览: 20
好的,我可以给您提供一个简单的图书管理系统的参考实现,使用JSP和SQL实现。
首先,数据库需要创建一个名为“books”的表,包含以下字段:
- id: 图书ID,自增长整数
- name: 图书名称,字符串
- author: 作者,字符串
- publisher: 出版社,字符串
- publishDate: 出版日期,日期类型
- price: 价格,浮点数类型
接下来,我们可以创建以下JSP页面来实现图书管理系统:
1. index.jsp:系统首页,包含“添加图书”、“查看图书”和“退出系统”三个链接。
```jsp
<html>
<head>
<title>图书管理系统</title>
</head>
<body>
<h1>图书管理系统</h1>
<a href="add.jsp">添加图书</a><br>
<a href="list.jsp">查看图书</a><br>
<a href="logout.jsp">退出系统</a>
</body>
</html>
```
2. add.jsp:添加图书页面,包含一个表单,可以输入图书信息。
```jsp
<html>
<head>
<title>添加图书</title>
</head>
<body>
<h1>添加图书</h1>
<form action="add.do" method="post">
<label>图书名称:</label><input type="text" name="name"><br>
<label>作者:</label><input type="text" name="author"><br>
<label>出版社:</label><input type="text" name="publisher"><br>
<label>出版日期:</label><input type="text" name="publishDate"><br>
<label>价格:</label><input type="text" name="price"><br>
<input type="submit" value="添加">
</form>
</body>
</html>
```
3. add.do:添加图书处理页面,将表单提交到此页面,将表单数据插入到数据库中,并跳转到“查看图书”页面。
```jsp
<%@ page import="java.sql.*" %>
<%
String name = request.getParameter("name");
String author = request.getParameter("author");
String publisher = request.getParameter("publisher");
String publishDate = request.getParameter("publishDate");
String price = request.getParameter("price");
Connection conn = null;
PreparedStatement stmt = null;
try {
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/test", "root", "123456");
String sql = "insert into books(name, author, publisher, publishDate, price) values (?, ?, ?, ?, ?)";
stmt = conn.prepareStatement(sql);
stmt.setString(1, name);
stmt.setString(2, author);
stmt.setString(3, publisher);
stmt.setString(4, publishDate);
stmt.setFloat(5, Float.parseFloat(price));
stmt.executeUpdate();
response.sendRedirect("list.jsp");
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (stmt != null) stmt.close();
if (conn != null) conn.close();
} catch (Exception e) {}
}
%>
```
4. list.jsp:查看图书页面,从数据库中查询所有图书信息,并显示在表格中。
```jsp
<%@ page import="java.sql.*" %>
<html>
<head>
<title>查看图书</title>
</head>
<body>
<h1>查看图书</h1>
<table border="1">
<tr>
<th>ID</th>
<th>图书名称</th>
<th>作者</th>
<th>出版社</th>
<th>出版日期</th>
<th>价格</th>
</tr>
<%
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/test", "root", "123456");
String sql = "select * from books";
stmt = conn.createStatement();
rs = stmt.executeQuery(sql);
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
String author = rs.getString("author");
String publisher = rs.getString("publisher");
Date publishDate = rs.getDate("publishDate");
float price = rs.getFloat("price");
%>
<tr>
<td><%= id %></td>
<td><%= name %></td>
<td><%= author %></td>
<td><%= publisher %></td>
<td><%= publishDate %></td>
<td><%= price %></td>
</tr>
<%
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (rs != null) rs.close();
if (stmt != null) stmt.close();
if (conn != null) conn.close();
} catch (Exception e) {}
}
%>
</table>
</body>
</html>
```
5. logout.jsp:退出系统页面,清除session,并跳转到系统首页。
```jsp
<%
session.invalidate();
response.sendRedirect("index.jsp");
%>
```
以上就是一个简单的图书管理系统的JSP和SQL实现,供您参考。
相关推荐
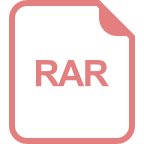
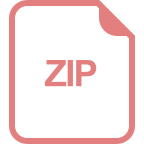
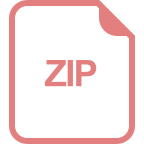














