请实现代码,指定某个usb 设备只读,并允许C# 程式调用
时间: 2024-03-21 22:39:01 浏览: 179
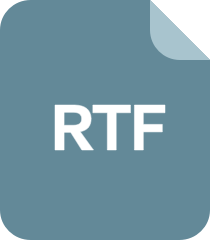
C#实现USB接口的程序代码

以下是一份 C# 的示例代码,通过调用 libusb 库实现控制指定 USB 设备只读:
```csharp
using System;
using System.Runtime.InteropServices;
namespace USBDeviceReadOnlyControl {
class Program {
// 定义 libusb 库的函数
[DllImport("libusb-1.0.dll", CallingConvention = CallingConvention.Cdecl)]
public static extern int libusb_init(IntPtr ctx);
[DllImport("libusb-1.0.dll", CallingConvention = CallingConvention.Cdecl)]
public static extern int libusb_exit(IntPtr ctx);
[DllImport("libusb-1.0.dll", CallingConvention = CallingConvention.Cdecl)]
public static extern int libusb_get_device_list(IntPtr ctx, ref IntPtr list);
[DllImport("libusb-1.0.dll", CallingConvention = CallingConvention.Cdecl)]
public static extern void libusb_free_device_list(IntPtr list, int unref_devices);
[DllImport("libusb-1.0.dll", CallingConvention = CallingConvention.Cdecl)]
public static extern int libusb_get_device_descriptor(IntPtr dev, ref libusb_device_descriptor desc);
[DllImport("libusb-1.0.dll", CallingConvention = CallingConvention.Cdecl)]
public static extern int libusb_open(IntPtr dev, ref IntPtr handle);
[DllImport("libusb-1.0.dll", CallingConvention = CallingConvention.Cdecl)]
public static extern void libusb_close(IntPtr handle);
[DllImport("libusb-1.0.dll", CallingConvention = CallingConvention.Cdecl)]
public static extern int libusb_control_transfer(IntPtr handle, byte request_type, byte request, ushort value, ushort index, byte[] data, ushort length, uint timeout);
// 定义 libusb 库的结构体
[StructLayout(LayoutKind.Sequential)]
public struct libusb_device_descriptor {
public byte bLength;
public byte bDescriptorType;
public ushort bcdUSB;
public byte bDeviceClass;
public byte bDeviceSubClass;
public byte bDeviceProtocol;
public byte bMaxPacketSize0;
public ushort idVendor;
public ushort idProduct;
public ushort bcdDevice;
public byte iManufacturer;
public byte iProduct;
public byte iSerialNumber;
public byte bNumConfigurations;
}
// 控制 USB 设备只读的函数
public static int MakeUSBDeviceReadOnly(IntPtr dev_handle) {
int r = 0;
byte[] buffer = new byte[8];
// 发送命令给 USB 设备
buffer[0] = 0x0F; // 假设 0x0F 是只读命令
r = libusb_control_transfer(dev_handle, 0x40, 0x00, 0x00, 0x00, buffer, (ushort)buffer.Length, 1000);
if (r < 0) {
// 控制失败,返回错误码
return r;
}
// 控制成功,返回 0
return 0;
}
// 获取指定 Vendor ID 和 Product ID 的 USB 设备
public static IntPtr GetUSBDeviceByVIDPID(IntPtr list, ushort vendor_id, ushort product_id) {
IntPtr dev = IntPtr.Zero;
IntPtr dev_handle = IntPtr.Zero;
int r = 0;
// 遍历 USB 设备列表,查找指定 Vendor ID 和 Product ID 的设备
for (int i = 0; ; i++) {
IntPtr p = Marshal.ReadIntPtr(list, i * IntPtr.Size);
if (p == IntPtr.Zero) {
break;
}
libusb_device_descriptor desc = new libusb_device_descriptor();
r = libusb_get_device_descriptor(p, ref desc);
if (r < 0) {
continue;
}
if (desc.idVendor == vendor_id && desc.idProduct == product_id) {
// 打开设备
r = libusb_open(p, ref dev_handle);
if (r < 0) {
continue;
}
// 控制设备只读
r = MakeUSBDeviceReadOnly(dev_handle);
if (r < 0) {
continue;
}
// 找到了指定设备,返回设备句柄
dev = dev_handle;
break;
}
}
return dev;
}
// 示例代码
static void Main(string[] args) {
int r = 0;
IntPtr ctx = IntPtr.Zero;
IntPtr list = IntPtr.Zero;
IntPtr dev_handle = IntPtr.Zero;
// 初始化 libusb 库
r = libusb_init(ref ctx);
if (r < 0) {
// 初始化失败,返回错误码
Console.WriteLine("Failed to initialize libusb: {0}", r);
return;
}
// 获取 USB 设备列表
r = libusb_get_device_list(ctx, ref list);
if (r < 0) {
// 获取失败,返回错误码
Console.WriteLine("Failed to get USB device list: {0}", r);
libusb_exit(ctx);
return;
}
// 获取指定 Vendor ID 和 Product ID 的 USB 设备
ushort vendor_id = 0x1234; // 假设 Vendor ID 是 0x1234
ushort product_id = 0x5678; // 假设 Product ID 是 0x5678
dev_handle = GetUSBDeviceByVIDPID(list, vendor_id, product_id);
if (dev_handle == IntPtr.Zero) {
// 没有找到指定设备,返回错误码
Console.WriteLine("Failed to find USB device: {0:X4}:{1:X4}", vendor_id, product_id);
libusb_free_device_list(list, 1);
libusb_exit(ctx);
return;
}
// 关闭 USB 设备
libusb_close(dev_handle);
// 释放 USB 设备列表和 libusb 资源
libusb_free_device_list(list, 1);
libusb_exit(ctx);
// 控制成功,输出提示信息
Console.WriteLine("USB device {0:X4}:{1:X4} is now read-only.", vendor_id, product_id);
}
}
}
```
以上代码中,`GetUSBDeviceByVIDPID` 函数可以根据 Vendor ID 和 Product ID 获取指定的 USB 设备,并控制其只读。在实际使用时,需要将 Vendor ID 和 Product ID 修改为实际的值。另外,需要将 libusb 库的 DLL 文件拷贝到程序运行目录下。
阅读全文
相关推荐
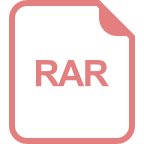
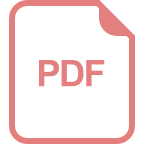
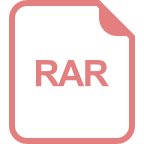
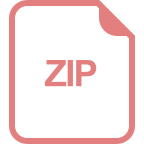
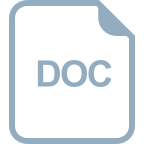
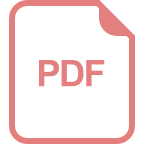
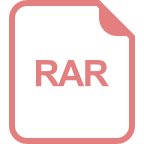
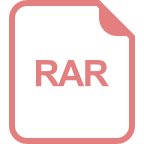
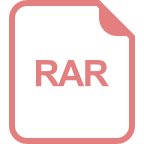
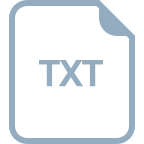
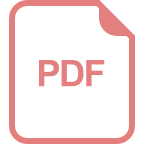
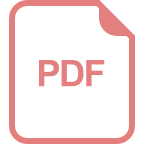
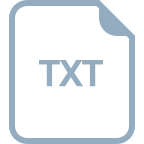
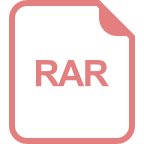