C++使用openssl对Base64字符串进行解码
时间: 2023-12-24 18:02:37 浏览: 84
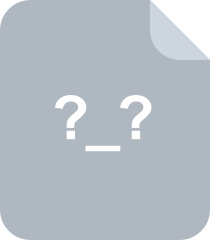
C++关于base64编码解码
你可以使用openssl库中的函数来解码Base64字符串。具体步骤如下:
1. 引入openssl库头文件和命名空间:
```c++
#include <openssl/bio.h>
#include <openssl/evp.h>
#include <openssl/buffer.h>
using namespace std;
```
2. 定义解码函数:
```c++
string base64_decode(const string& input) {
BIO *bio, *b64;
char *buffer = new char[input.length()];
memset(buffer, 0, input.length());
b64 = BIO_new(BIO_f_base64());
BIO_set_flags(b64, BIO_FLAGS_BASE64_NO_NL);
bio = BIO_new_mem_buf(input.c_str(), input.length());
bio = BIO_push(b64, bio);
BIO_read(bio, buffer, input.length());
BIO_free_all(bio);
string result(buffer);
delete[] buffer;
return result;
}
```
3. 调用解码函数:
```c++
string encodedString = "SGVsbG8gV29ybGQh";
string decodedString = base64_decode(encodedString);
cout << decodedString << endl;
```
输出结果为:Hello World!
注意:解码后的字符串不一定是可读的文本,因为Base64编码可以将二进制数据转换为文本格式。如果你需要解码的是文本数据,那么解码后的字符串应该是可读的文本。
阅读全文
相关推荐
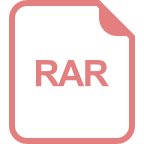

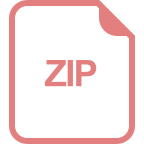
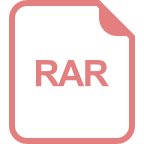
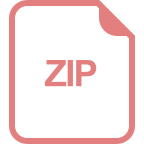
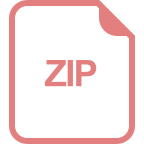
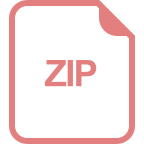
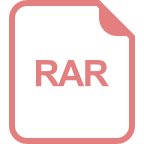
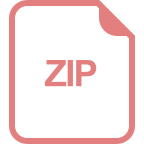
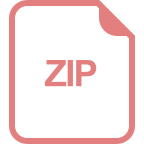
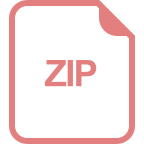
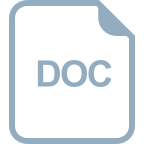
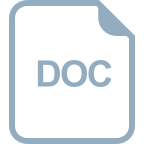

