#include <stdio.h> int main { int a,b,c; c=a scanf("%d,%d",&a,&b); if(a&&b) printf("c=%d"\n,c); else printf("c=%d"\n,c--); return 0; }有什么错误
时间: 2024-03-07 17:48:34 浏览: 87
这段代码存在多个错误,包括但不限于:
1. `main` 函数的参数列表应该是 `(void)` 或者 `(int argc, char* argv[])`,你的代码缺少了括号和参数类型声明。
2. 在 `c=a` 语句之前,`a` 和 `b` 还没有被初始化,这样赋值是没有意义的,也是未定义行为。
3. 在 `printf` 函数中,字符串常量应该用双引号括起来,而不是单引号。
4. 在 `printf` 函数中,变量 `c` 没有被初始化,输出的值是未定义的。
5. 在 `else` 语句中,应该是 `c--` 而不是 `c\n`。
正确的代码应该是这样的:
```c
#include <stdio.h>
int main(void) {
int a, b, c = 0;
scanf("%d,%d", &a, &b);
if (a && b) {
c = a;
} else {
c = b;
}
printf("c=%d\n", c);
return 0;
}
```
在这个修正后的代码中,我们初始化了 `c` 的值为 0,然后根据输入的 `a` 和 `b` 的值,将 `c` 赋值为其中一个非零的值。然后输出 `c` 的值。
相关问题
#include+<stdio.h> int+main()+{ int+n,+i,+a,+b1,+b2,+max,+min,+c+; int+j+=+2; scanf("%d",+&n);
这是一个C语言程序,主要功能是读入一个整数n,然后进行一些计算。具体来说,程序中定义了一些整型变量n、i、a、b1、b2、max、min和c,然后通过scanf函数读入一个整数n。接下来程序中使用了一些运算符和控制语句,但是缺少了完整的代码,无法确定程序的具体功能。需要提供更多的代码或者问题描述才能回答你的问题。
以下程序对输入的两个整数,按从小到大的顺序输出。请补全程序。 #include<stdio.h> int main() { int a,b,t; scanf("%d,%d",&a,&b); if( 2 分 ) { t=a; 3 分 //两条语句; } printf("%d,%d",a,b); return 0; }
以下是补全后的程序:
```c
#include <stdio.h>
int main() {
int a, b, t;
scanf("%d,%d", &a, &b);
if (a > b) { // 判断a是否大于b
t = a;
a = b;
b = t; // 交换a和b的值
}
printf("%d,%d", a, b);
return 0;
}
```
算法思路:
1. 从标准输入中读入两个整数a和b;
2. 判断a是否大于b,若是则交换a和b的值;
3. 输出a和b的值。
阅读全文
相关推荐
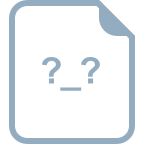
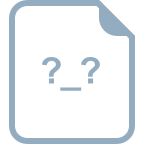















