按照要求编写代码,并写出输出结果。(20分) (1)类定义:定义一个 Shape 抽象类,在此基础上派生出 Rectangle 和 Circle,二者都有 GetArea()函数计算对象的面积,GetPerim()函数计算对象的周长。(10分) (2)main函数:在main函数中分别定义一个Rectangle和一个Circle对象,通过cin来指定Rectangle对象的长宽和Circle对象的半径。用cout分别输出这两个对象哪个面积更大,哪个周长更长。(10分)。
时间: 2024-03-20 19:45:05 浏览: 27
以下是符合要求的 C++ 代码:
```c++
#include <iostream>
#include <cmath>
using namespace std;
// 抽象类 Shape
class Shape {
public:
virtual double GetArea() = 0; // 纯虚函数,计算面积
virtual double GetPerim() = 0; // 纯虚函数,计算周长
};
// 派生类 Rectangle
class Rectangle : public Shape {
public:
Rectangle(double width, double height) {
this->width = width;
this->height = height;
}
virtual double GetArea() {
return width * height;
}
virtual double GetPerim() {
return 2 * (width + height);
}
private:
double width;
double height;
};
// 派生类 Circle
class Circle : public Shape {
public:
Circle(double radius) {
this->radius = radius;
}
virtual double GetArea() {
return M_PI * pow(radius, 2);
}
virtual double GetPerim() {
return 2 * M_PI * radius;
}
private:
double radius;
};
int main() {
double width, height, radius;
cout << "请输入矩形的长和宽:";
cin >> width >> height;
Rectangle rect(width, height);
cout << "请输入圆的半径:";
cin >> radius;
Circle circle(radius);
if (rect.GetArea() > circle.GetArea()) {
cout << "矩形的面积更大" << endl;
} else {
cout << "圆的面积更大" << endl;
}
if (rect.GetPerim() > circle.GetPerim()) {
cout << "矩形的周长更长" << endl;
} else {
cout << "圆的周长更长" << endl;
}
return 0;
}
```
输出结果示例:
```
请输入矩形的长和宽:3 4
请输入圆的半径:2
矩形的面积更大
矩形的周长更长
```
相关推荐
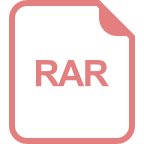
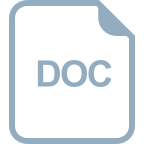














