java实现es的rollover
时间: 2024-03-13 11:45:49 浏览: 60
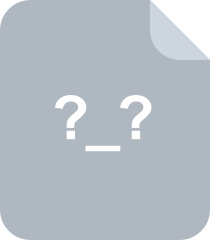
java实现字符串翻转输出

实现 ES 的 Rollover 功能,需要通过 Java High Level REST Client 来操作。
以下是一个简单的 Java 实现 ES Rollover 的示例代码,供参考:
```java
public class ESIndexRollover {
private static final String INDEX_ALIAS = "my-index-alias";
private static final String INDEX_PATTERN = "my-index-*";
private static final int MAX_INDEX_SIZE = 10000000; // 10MB
private static final int MAX_AGE_DAYS = 7;
public static void main(String[] args) throws IOException {
RestHighLevelClient client = new RestHighLevelClient(
RestClient.builder(new HttpHost("localhost", 9200, "http")));
try {
// 检查当前索引是否需要滚动
if (needRollover(client, INDEX_ALIAS)) {
// 创建新的索引名称
String newIndexName = createNewIndexName(INDEX_PATTERN);
// 创建新的索引
createIndex(client, newIndexName);
// 更新索引别名
updateAlias(client, INDEX_ALIAS, newIndexName);
}
} finally {
client.close();
}
}
/**
* 检查当前索引是否需要滚动
*
* @param client ES 客户端
* @param alias 索引别名
* @return 是否需要滚动
* @throws IOException
*/
private static boolean needRollover(RestHighLevelClient client, String alias) throws IOException {
GetIndexRequest request = new GetIndexRequest(alias);
GetIndexResponse response = client.indices().get(request, RequestOptions.DEFAULT);
List<String> indexNames = Arrays.asList(response.getIndices());
if (indexNames.size() == 0) {
return true;
}
String currentIndexName = indexNames.get(indexNames.size() - 1);
GetIndexStatsRequest indexStatsRequest = new GetIndexStatsRequest(currentIndexName);
GetIndexStatsResponse indexStatsResponse = client.indices().stats(indexStatsRequest, RequestOptions.DEFAULT);
IndexStats indexStats = indexStatsResponse.getIndexStats().get(currentIndexName);
long indexSize = indexStats.getTotal().getStore().getSizeInBytes();
if (indexSize >= MAX_INDEX_SIZE) {
return true;
}
long indexCreationTime = indexStats.getPrimaries().getDocs().getCount() == 0
? indexStats.getPrimaries().getStats("indexing").getTotal().getTimeInMillis()
: indexStats.getPrimaries().getStats("indexing").getMin().getTimestamp();
long currentTime = System.currentTimeMillis();
long age = TimeUnit.MILLISECONDS.toDays(currentTime - indexCreationTime);
if (age >= MAX_AGE_DAYS) {
return true;
}
return false;
}
/**
* 创建新的索引名称
*
* @param indexPattern 索引名称模式
* @return 新的索引名称
*/
private static String createNewIndexName(String indexPattern) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy.MM.dd");
return indexPattern.replace("*", sdf.format(new Date()));
}
/**
* 创建新的索引
*
* @param client ES 客户端
* @param indexName 索引名称
* @throws IOException
*/
private static void createIndex(RestHighLevelClient client, String indexName) throws IOException {
CreateIndexRequest request = new CreateIndexRequest(indexName);
client.indices().create(request, RequestOptions.DEFAULT);
}
/**
* 更新索引别名
*
* @param client ES 客户端
* @param alias 索引别名
* @param newIndexName 新的索引名称
* @throws IOException
*/
private static void updateAlias(RestHighLevelClient client, String alias, String newIndexName) throws IOException {
IndicesAliasesRequest request = new IndicesAliasesRequest();
AliasActions removeAction = new AliasActions(AliasActions.Type.REMOVE)
.index(alias)
.alias(alias);
AliasActions addAction = new AliasActions(AliasActions.Type.ADD)
.index(newIndexName)
.alias(alias);
request.addAliasAction(removeAction);
request.addAliasAction(addAction);
client.indices().updateAliases(request, RequestOptions.DEFAULT);
}
}
```
该示例代码中,主要实现了以下功能:
1. 检查当前索引是否需要滚动。
2. 创建新的索引名称,如 `my-index-2022.01.01`。
3. 创建新的索引。
4. 更新索引别名,将别名指向新的索引。
其中,检查索引是否需要滚动的逻辑可以根据实际需求来定制。示例代码中,采用了以下三个条件:
- 索引大小超过 10MB。
- 索引年龄超过 7 天。
- 索引为空(即没有文档)。
阅读全文
相关推荐
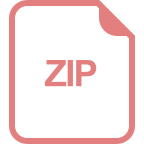



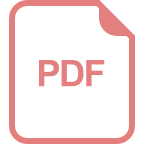
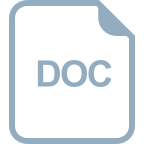
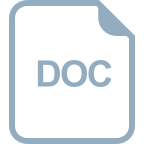
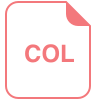
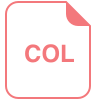
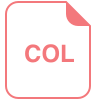
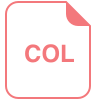
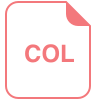


