写一段stm32控制三相无刷电机的驱动代码
时间: 2023-12-23 15:05:48 浏览: 230
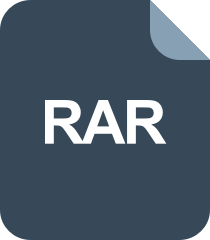
STM32F103三相无刷电机按键调速程序最终版.rar

以下是一个基本的STM32控制三相无刷电机的驱动代码示例。
```
#include "stm32f4xx.h"
// 定义PWM输出引脚
#define PWM_PIN_A GPIO_Pin_0
#define PWM_PIN_B GPIO_Pin_1
#define PWM_PIN_C GPIO_Pin_2
// 定义相电流采样引脚
#define CURRENT_PIN_A GPIO_Pin_3
#define CURRENT_PIN_B GPIO_Pin_4
#define CURRENT_PIN_C GPIO_Pin_5
// 定义PWM频率和占空比
#define PWM_FREQUENCY 20000
#define PWM_DUTY_CYCLE 50
// 定义电机转速和电压常数
#define MOTOR_SPEED_CONSTANT 10
#define MOTOR_VOLTAGE_CONSTANT 5
// 定义PID参数
#define KP 1.0
#define KI 0.0
#define KD 0.0
// 定义电机状态
typedef enum {
MOTOR_STOPPED,
MOTOR_STARTING,
MOTOR_RUNNING
} MotorState;
// 初始化PWM输出引脚
void init_pwm_pins(void) {
GPIO_InitTypeDef GPIO_InitStruct;
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
GPIO_StructInit(&GPIO_InitStruct);
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStruct.GPIO_Pin = PWM_PIN_A | PWM_PIN_B | PWM_PIN_C;
GPIO_InitStruct.GPIO_OType = GPIO_OType_PP;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_100MHz;
GPIO_Init(GPIOA, &GPIO_InitStruct);
GPIO_PinAFConfig(GPIOA, GPIO_PinSource0, GPIO_AF_TIM1);
GPIO_PinAFConfig(GPIOA, GPIO_PinSource1, GPIO_AF_TIM1);
GPIO_PinAFConfig(GPIOA, GPIO_PinSource2, GPIO_AF_TIM1);
}
// 初始化相电流采样引脚
void init_current_pins(void) {
GPIO_InitTypeDef GPIO_InitStruct;
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
GPIO_StructInit(&GPIO_InitStruct);
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AN;
GPIO_InitStruct.GPIO_Pin = CURRENT_PIN_A | CURRENT_PIN_B | CURRENT_PIN_C;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(GPIOA, &GPIO_InitStruct);
}
// 初始化PWM定时器
void init_pwm_timer(void) {
TIM_TimeBaseInitTypeDef TIM_InitStruct;
TIM_OCInitTypeDef TIM_OC_InitStruct;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_TIM1, ENABLE);
TIM_InitStruct.TIM_Prescaler = ((SystemCoreClock / 2) / PWM_FREQUENCY) - 1;
TIM_InitStruct.TIM_Period = (100 / PWM_DUTY_CYCLE) - 1;
TIM_InitStruct.TIM_ClockDivision = TIM_CKD_DIV1;
TIM_InitStruct.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseInit(TIM1, &TIM_InitStruct);
TIM_OC_InitStruct.TIM_OCMode = TIM_OCMode_PWM1;
TIM_OC_InitStruct.TIM_OutputState = TIM_OutputState_Enable;
TIM_OC_InitStruct.TIM_Pulse = 0;
TIM_OC_InitStruct.TIM_OCPolarity = TIM_OCPolarity_High;
TIM_OC1Init(TIM1, &TIM_OC_InitStruct);
TIM_OC1PreloadConfig(TIM1, TIM_OCPreload_Enable);
TIM_OC_InitStruct.TIM_OCMode = TIM_OCMode_PWM1;
TIM_OC_InitStruct.TIM_OutputState = TIM_OutputState_Enable;
TIM_OC_InitStruct.TIM_Pulse = 0;
TIM_OC_InitStruct.TIM_OCPolarity = TIM_OCPolarity_High;
TIM_OC2Init(TIM1, &TIM_OC_InitStruct);
TIM_OC2PreloadConfig(TIM1, TIM_OCPreload_Enable);
TIM_OC_InitStruct.TIM_OCMode = TIM_OCMode_PWM1;
TIM_OC_InitStruct.TIM_OutputState = TIM_OutputState_Enable;
TIM_OC_InitStruct.TIM_Pulse = 0;
TIM_OC_InitStruct.TIM_OCPolarity = TIM_OCPolarity_High;
TIM_OC3Init(TIM1, &TIM_OC_InitStruct);
TIM_OC3PreloadConfig(TIM1, TIM_OCPreload_Enable);
TIM_Cmd(TIM1, ENABLE);
}
// 初始化ADC采样器
void init_adc(void) {
ADC_InitTypeDef ADC_InitStruct;
ADC_CommonInitTypeDef ADC_CommonInitStruct;
GPIO_InitTypeDef GPIO_InitStruct;
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_ADC1, ENABLE);
GPIO_StructInit(&GPIO_InitStruct);
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AN;
GPIO_InitStruct.GPIO_Pin = CURRENT_PIN_A | CURRENT_PIN_B | CURRENT_PIN_C;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(GPIOA, &GPIO_InitStruct);
ADC_CommonInitStruct.ADC_Mode = ADC_Mode_Independent;
ADC_CommonInitStruct.ADC_Prescaler = ADC_Prescaler_Div4;
ADC_CommonInitStruct.ADC_DMAAccessMode = ADC_DMAAccessMode_Disabled;
ADC_CommonInitStruct.ADC_TwoSamplingDelay = ADC_TwoSamplingDelay_5Cycles;
ADC_CommonInit(&ADC_CommonInitStruct);
ADC_StructInit(&ADC_InitStruct);
ADC_InitStruct.ADC_Resolution = ADC_Resolution_12b;
ADC_InitStruct.ADC_ScanConvMode = DISABLE;
ADC_InitStruct.ADC_ContinuousConvMode = ENABLE;
ADC_InitStruct.ADC_ExternalTrigConvEdge = ADC_ExternalTrigConvEdge_None;
ADC_InitStruct.ADC_DataAlign = ADC_DataAlign_Right;
ADC_InitStruct.ADC_NbrOfConversion = 1;
ADC_Init(ADC1, &ADC_InitStruct);
ADC_Cmd(ADC1, ENABLE);
}
// 读取相电流值
uint16_t read_current(uint16_t pin) {
ADC_RegularChannelConfig(ADC1, pin, 1, ADC_SampleTime_56Cycles);
ADC_SoftwareStartConv(ADC1);
while (ADC_GetFlagStatus(ADC1, ADC_FLAG_EOC) == RESET);
return ADC_GetConversionValue(ADC1);
}
// 计算电机转速
float calculate_speed(uint16_t current_a, uint16_t current_b, uint16_t current_c) {
float total_current = (float)(current_a + current_b + current_c) / 3.0;
return total_current * MOTOR_SPEED_CONSTANT;
}
// 计算电机电压
float calculate_voltage(float speed) {
return speed * MOTOR_VOLTAGE_CONSTANT;
}
// 控制电机转速
void control_motor(float setpoint_speed) {
static MotorState state = MOTOR_STOPPED;
static float current_speed = 0.0;
static float last_error = 0.0;
static float integral = 0.0;
uint16_t current_a = read_current(CURRENT_PIN_A);
uint16_t current_b = read_current(CURRENT_PIN_B);
uint16_t current_c = read_current(CURRENT_PIN_C);
float actual_speed = calculate_speed(current_a, current_b, current_c);
float error = setpoint_speed - actual_speed;
integral += error;
float derivative = error - last_error;
last_error = error;
float output = KP * error + KI * integral + KD * derivative;
if (state == MOTOR_STOPPED) {
if (setpoint_speed > 0.0) {
state = MOTOR_STARTING;
GPIO_ResetBits(GPIOA, PWM_PIN_A | PWM_PIN_B | PWM_PIN_C);
TIM_SetCompare1(TIM1, PWM_DUTY_CYCLE);
TIM_SetCompare2(TIM1, PWM_DUTY_CYCLE);
TIM_SetCompare3(TIM1, PWM_DUTY_CYCLE);
TIM_Cmd(TIM1, ENABLE);
}
} else if (state == MOTOR_STARTING) {
if (actual_speed > 0.1) {
state = MOTOR_RUNNING;
}
} else if (state == MOTOR_RUNNING) {
if (setpoint_speed == 0.0) {
state = MOTOR_STOPPED;
GPIO_ResetBits(GPIOA, PWM_PIN_A | PWM_PIN_B | PWM_PIN_C);
TIM_Cmd(TIM1, DISABLE);
} else {
float voltage = calculate_voltage(output);
TIM_SetCompare1(TIM1, PWM_DUTY_CYCLE * voltage / 100.0);
TIM_SetCompare2(TIM1, PWM_DUTY_CYCLE * voltage / 100.0);
TIM_SetCompare3(TIM1, PWM_DUTY_CYCLE * voltage / 100.0);
}
}
}
int main(void) {
init_pwm_pins();
init_current_pins();
init_pwm_timer();
init_adc();
while(1) {
control_motor(100.0);
}
}
```
该代码使用STM32的PWM输出引脚和ADC采样器控制三相无刷电机。代码中实现了PID控制,以控制电机的转速。控制电机的转速需要读取三相电流的值,并计算电机的转速和电压值。代码中还实现了电机的启动和停止功能。
阅读全文
相关推荐
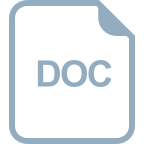
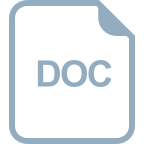
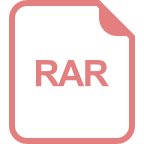
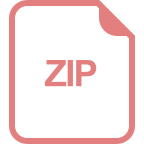
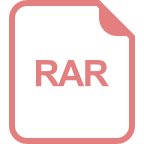
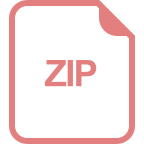
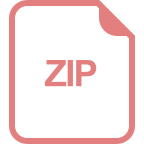
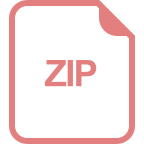
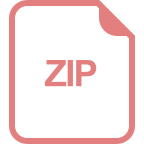
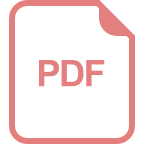
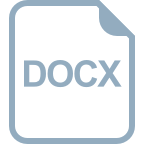
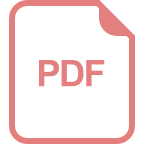
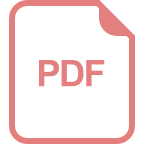
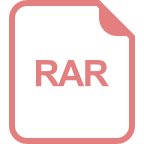
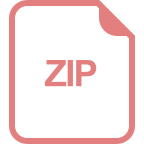
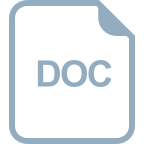
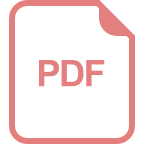
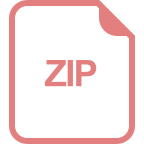