Java jsoup web爬取京东图片 代码
时间: 2023-03-19 22:25:00 浏览: 122
以下是使用Java和jsoup库从京东网站爬取商品图片的代码示例:
```java
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.util.ArrayList;
import java.util.List;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
public class JdImageCrawler {
public static void main(String[] args) throws IOException {
String url = "https://search.jd.com/Search?keyword=手机";
List<String> imageUrls = getJdImageUrls(url);
downloadImages(imageUrls);
}
/**
* 从京东搜索结果页面获取商品图片链接
* @param url 京东搜索结果页面的URL
* @return 商品图片链接列表
* @throws IOException
*/
public static List<String> getJdImageUrls(String url) throws IOException {
List<String> imageUrls = new ArrayList<>();
Document doc = Jsoup.connect(url).get();
Elements elements = doc.select(".gl-item .p-img img");
for (Element element : elements) {
String imageUrl = element.attr("data-lazy-img");
if (imageUrl == null || imageUrl.isEmpty()) {
imageUrl = element.attr("src");
}
imageUrls.add(imageUrl.replace("/n9/", "/n1/"));
}
return imageUrls;
}
/**
* 下载图片到本地
* @param imageUrls 商品图片链接列表
* @throws IOException
*/
public static void downloadImages(List<String> imageUrls) throws IOException {
for (String imageUrl : imageUrls) {
URL url = new URL(imageUrl);
InputStream is = url.openStream();
String fileName = imageUrl.substring(imageUrl.lastIndexOf("/") + 1);
byte[] bytes = new byte[1024];
int len;
try (FileOutputStream fos = new FileOutputStream(fileName)) {
while ((len = is.read(bytes)) != -1) {
fos.write(bytes, 0, len);
}
}
}
}
}
```
这个代码示例使用jsoup库从京东搜索结果页面获取商品图片链接,并使用Java标准库下载这些图片到本地。注意,这个示例代码并没有处理异常情况,实际应用中需要加入更多的错误处理和异常处理代码。
相关推荐
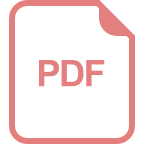
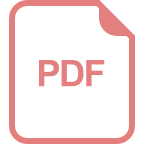














