单片机按键按下数码管显示值+1,数码管在0-9间循环的C语言程序怎么写
时间: 2024-02-18 17:06:17 浏览: 37
好的,以下是一个简单的C语言程序,实现了按键按下数码管显示值+1,数码管在0-9间循环显示的功能。假设我们使用的是STM32单片机,其中PA0引脚接了一个按键,PB0~PB3引脚接了一个4位共阳数码管。代码如下:
```c
#include "stm32f10x.h" // 包含STM32标准库
#include "stdio.h" // 包含标准输入输出库
int main(void)
{
GPIO_InitTypeDef GPIO_InitStructure; // 定义GPIO结构体
uint8_t value = 0; // 定义初始值为0
// 打开GPIOA和GPIOB的时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_GPIOB, ENABLE);
// 配置PA0为输入模式,上拉输入
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置PB0~PB3为推挽输出模式
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_2 | GPIO_Pin_3;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
while(1)
{
if(GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_0) == 0) // 判断PA0是否被按下
{
value++; // 值+1
if(value > 9) // 如果值超过9,回到0
{
value = 0;
}
// 将值写入数码管
GPIO_WriteBit(GPIOB, GPIO_Pin_0, (value & 0x01) ? Bit_SET : Bit_RESET);
GPIO_WriteBit(GPIOB, GPIO_Pin_1, (value & 0x02) ? Bit_SET : Bit_RESET);
GPIO_WriteBit(GPIOB, GPIO_Pin_2, (value & 0x04) ? Bit_SET : Bit_RESET);
GPIO_WriteBit(GPIOB, GPIO_Pin_3, (value & 0x08) ? Bit_SET : Bit_RESET);
}
}
}
```
以上代码中,首先我们打开了GPIOA和GPIOB的时钟,并对PA0和PB0~PB3进行了配置。然后在while循环中,我们不断地检测PA0是否被按下,如果按下了就将value值+1,然后根据value值将数码管显示相应的数字。需要注意的是,我们在将值写入数码管时使用了位运算,这样可以将value值的二进制表示的每一位与对应的引脚相连,从而实现了将数字显示在数码管上的功能。
相关推荐
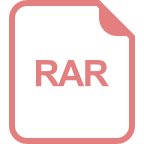
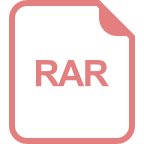
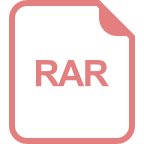














