下一步,创建控制器类com.javaee.ex12.controller.StudentController, 并在其中添加两个方法,分别用于页面跳转和添加学生信息。 下一步,在“WEB-INF/pages/student目录中,创建add_student.jsp文件,提交选修课程。选修课程包括选课信息,学号,年龄,课程列表。课程列表中包含大学英语,高等数学,程序设计基础,计算机网络。下一步,在“WEB-INF/pages/student”目录中,创建student_info.jsp文件,用于显示请求区域中的数据,给出对应代码。下一步,在pom.xml中,添加jstl依赖。
时间: 2023-07-15 20:11:51 浏览: 129
好的,下面是对应的代码:
StudentController.java
```java
package com.javaee.ex12.controller;
import javax.servlet.http.HttpServletRequest;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
@Controller
@RequestMapping("/student")
public class StudentController {
@RequestMapping(value = "/add", method = RequestMethod.GET)
public String addStudentPage(ModelMap model) {
return "add_student";
}
@RequestMapping(value = "/add", method = RequestMethod.POST)
public String addStudent(HttpServletRequest request, ModelMap model) {
String id = request.getParameter("id");
String age = request.getParameter("age");
String[] courses = request.getParameterValues("courses");
// 保存学生信息和选课信息
model.addAttribute("id", id);
model.addAttribute("age", age);
model.addAttribute("courses", courses);
return "student_info";
}
}
```
add_student.jsp
```html
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<html>
<head>
<title>Add Student Page</title>
</head>
<body>
<h2>Add Student</h2>
<form action="${pageContext.request.contextPath}/student/add" method="post">
<table>
<tr>
<td>Id:</td>
<td><input type="text" name="id"></td>
</tr>
<tr>
<td>Age:</td>
<td><input type="text" name="age"></td>
</tr>
<tr>
<td>Courses:</td>
<td>
<input type="checkbox" name="courses" value="University English">University English<br>
<input type="checkbox" name="courses" value="Advanced Mathematics">Advanced Mathematics<br>
<input type="checkbox" name="courses" value="Programming Foundation">Programming Foundation<br>
<input type="checkbox" name="courses" value="Computer Network">Computer Network<br>
</td>
</tr>
<tr>
<td colspan="2"><input type="submit" value="Submit"></td>
</tr>
</table>
</form>
</body>
</html>
```
student_info.jsp
```html
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<html>
<head>
<title>Student Information</title>
</head>
<body>
<h2>Student Information</h2>
<table>
<tr>
<td>Id:</td>
<td>${id}</td>
</tr>
<tr>
<td>Age:</td>
<td>${age}</td>
</tr>
<tr>
<td>Courses:</td>
<td>
<c:forEach var="course" items="${courses}">
${course}<br>
</c:forEach>
</td>
</tr>
</table>
</body>
</html>
```
pom.xml
```xml
<dependencies>
<dependency>
<groupId>jstl</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
</dependency>
</dependencies>
```
阅读全文
相关推荐



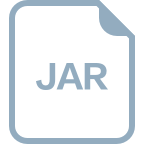


