写一个Java的校园的商品管理系统
时间: 2024-04-22 14:25:52 浏览: 17
好的,校园商品管理系统可以包含以下功能:
1. 商品管理:包括商品的添加、修改、删除和查询功能;
2. 库存管理:包括库存的入库、出库、盘点和查询功能;
3. 销售管理:包括销售的录入、统计和查询功能;
4. 用户管理:包括用户的注册、登录、修改密码和退出登录功能;
5. 数据统计:包括商品销售量、库存量和销售额的统计功能。
以下是一个简单的Java代码实现:
```
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Scanner;
public class CampusGoodsManagementSystem {
private static HashMap<Integer, Goods> goodsMap = new HashMap<>();
private static ArrayList<Inventory> inventoryList = new ArrayList<>();
private static ArrayList<Sale> saleList = new ArrayList<>();
private static ArrayList<User> userList = new ArrayList<>();
private static User currentUser = null;
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
boolean isExit = false;
while (!isExit) {
System.out.println("欢迎使用校园商品管理系统!");
System.out.println("1.用户登录");
System.out.println("2.用户注册");
System.out.println("3.商品管理");
System.out.println("4.库存管理");
System.out.println("5.销售管理");
System.out.println("6.数据统计");
System.out.println("0.退出系统");
System.out.print("请选择操作:");
int choice = scanner.nextInt();
switch (choice) {
case 1:
login();
break;
case 2:
register();
break;
case 3:
goodsManagement();
break;
case 4:
inventoryManagement();
break;
case 5:
saleManagement();
break;
case 6:
dataStatistics();
break;
case 0:
isExit = true;
break;
default:
System.out.println("输入错误,请重新选择!");
break;
}
}
System.out.println("谢谢使用校园商品管理系统!");
}
private static void login() {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入用户名:");
String username = scanner.nextLine();
System.out.print("请输入密码:");
String password = scanner.nextLine();
for (User user : userList) {
if (user.getUsername().equals(username) && user.getPassword().equals(password)) {
currentUser = user;
System.out.println("登录成功!");
return;
}
}
System.out.println("用户名或密码错误,登录失败!");
}
private static void register() {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入用户名:");
String username = scanner.nextLine();
System.out.print("请输入密码:");
String password = scanner.nextLine();
User user = new User(username, password);
userList.add(user);
System.out.println("注册成功!");
}
private static void goodsManagement() {
if (currentUser == null) {
System.out.println("请先登录!");
return;
}
Scanner scanner = new Scanner(System.in);
System.out.println("1.添加商品");
System.out.println("2.修改商品");
System.out.println("3.删除商品");
System.out.println("4.查询商品");
System.out.print("请选择操作:");
int choice = scanner.nextInt();
switch (choice) {
case 1:
System.out.print("请输入商品编号:");
int id = scanner.nextInt();
System.out.print("请输入商品名称:");
String name = scanner.nextLine();
System.out.print("请输入商品价格:");
double price = scanner.nextDouble();
Goods goods = new Goods(id, name, price);
goodsMap.put(id, goods);
System.out.println("添加成功!");
break;
case 2:
System.out.print("请输入要修改的商品编号:");
int idToUpdate = scanner.nextInt();
if (!goodsMap.containsKey(idToUpdate)) {
System.out.println("商品不存在!");
return;
}
Goods goodsToUpdate = goodsMap.get(idToUpdate);
System.out.print("请输入新的商品名称(留空表示不修改):");
String newName = scanner.nextLine();
if (!newName.isEmpty()) {
goodsToUpdate.setName(newName);
}
System.out.print("请输入新的商品价格(留空表示不修改):");
String newPriceStr = scanner.nextLine();
if (!newPriceStr.isEmpty()) {
double newPrice = Double.parseDouble(newPriceStr);
goodsToUpdate.setPrice(newPrice);
}
System.out.println("修改成功!");
break;
case 3:
System.out.print("请输入要删除的商品编号:");
int idToDelete = scanner.nextInt();
if (!goodsMap.containsKey(idToDelete)) {
System.out.println("商品不存在!");
return;
}
goodsMap.remove(idToDelete);
System.out.println("删除成功!");
break;
case 4:
System.out.print("请输入要查询的商品编号:");
int idToQuery = scanner.nextInt();
if (!goodsMap.containsKey(idToQuery)) {
System.out.println("商品不存在!");
return;
}
Goods goodsToQuery = goodsMap.get(idToQuery);
System.out.println("商品编号:" + goodsToQuery.getId());
System.out.println("商品名称:" + goodsToQuery.getName());
System.out.println("商品价格:" + goodsToQuery.getPrice());
break;
default:
System.out.println("输入错误,请重新选择!");
break;
}
}
private static void inventoryManagement() {
if (currentUser == null) {
System.out.println("请先登录!");
return;
}
Scanner scanner = new Scanner(System.in);
System.out.println("1.入库");
System.out.println("2.出库");
System.out.println("3.盘点");
System.out.println("4.查询库存");
System.out.print("请选择操作:");
int choice = scanner.nextInt();
switch (choice) {
case 1:
System.out.print("请输入商品编号:");
int id = scanner.nextInt();
if (!goodsMap.containsKey(id)) {
System.out.println("商品不存在!");
return;
}
System.out.print("请输入入库数量:");
int quantityIn = scanner.nextInt();
Inventory inventoryIn = new Inventory(id, quantityIn);
inventoryList.add(inventoryIn);
System.out.println("入库成功!");
break;
case 2:
System.out.print("请输入商品编号:");
int idOut = scanner.nextInt();
if (!goodsMap.containsKey(idOut)) {
System.out.println("商品不存在!");
return;
}
System.out.print("请输入出库数量:");
int quantityOut = scanner.nextInt();
if (!isInventoryEnough(idOut, quantityOut)) {
System.out.println("库存不足!");
return;
}
Inventory inventoryOut = new Inventory(idOut, -quantityOut);
inventoryList.add(inventoryOut);
System.out.println("出库成功!");
break;
case 3:
System.out.print("请输入商品编号:");
int idCheck = scanner.nextInt();
if (!goodsMap.containsKey(idCheck)) {
System.out.println("商品不存在!");
return;
}
int quantityCheck = getInventoryQuantity(idCheck);
System.out.println("商品编号:" + idCheck);
System.out.println("库存数量:" + quantityCheck);
break;
case 4:
System.out.println("商品编号\t库存数量");
for (Inventory inventory : inventoryList) {
int idQuery = inventory.getGoodsId();
int quantityQuery = getInventoryQuantity(idQuery);
System.out.println(idQuery + "\t" + quantityQuery);
}
break;
default:
System.out.println("输入错误,请重新选择!");
break;
}
}
private static boolean isInventoryEnough(int goodsId, int quantity) {
int currentQuantity = getInventoryQuantity(goodsId);
return currentQuantity >= quantity;
}
private static int getInventoryQuantity(int goodsId) {
int quantity = 0;
for (Inventory inventory : inventoryList) {
if (inventory.getGoodsId() == goodsId) {
quantity += inventory.getQuantity();
}
}
return quantity;
}
private static void saleManagement() {
if (currentUser == null) {
System.out.println("请先登录!");
return;
}
Scanner scanner = new Scanner(System.in);
System.out.print("请输入商品编号:");
int id = scanner.nextInt();
if (!goodsMap.containsKey(id)) {
System.out.println("商品不存在!");
return;
}
System.out.print("请输入销售数量:");
int quantity = scanner.nextInt();
if (!isInventoryEnough(id, quantity)) {
System.out.println("库存不足!");
return;
}
double price = goodsMap.get(id).getPrice();
double totalPrice = price * quantity;
Sale sale = new Sale(id, quantity, totalPrice);
saleList.add(sale);
Inventory inventory = new Inventory(id, -quantity);
inventoryList.add(inventory);
System.out.println("销售成功!");
}
private static void dataStatistics() {
if (currentUser == null) {
System.out.println("请先登录!");
return;
}
double totalSales = 0;
for (Sale sale : saleList) {
totalSales += sale.getTotalPrice();
}
System.out.println("商品销售总额:" + totalSales);
System.out.println("商品销售统计:");
System.out.println("商品编号\t销售数量\t销售额");
for (Sale sale : saleList) {
int id = sale.getGoodsId();
int quantity = sale.getQuantity();
double totalPrice = sale.getTotalPrice();
System.out.println(id + "\t" + quantity + "\t" + totalPrice);
}
}
private static class Goods {
private int id;
private String name;
private double price;
public Goods(int id, String name, double price) {
this.id = id;
this.name = name;
this.price = price;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
}
private static class Inventory {
private int goodsId;
private int quantity;
public Inventory(int goodsId, int quantity) {
this.goodsId = goodsId;
this.quantity = quantity;
}
public int getGoodsId() {
return goodsId;
}
public void setGoodsId(int goodsId) {
this.goodsId = goodsId;
}
public int getQuantity() {
return quantity;
}
public void setQuantity(int quantity) {
this.quantity = quantity;
}
}
private static class Sale {
private int goodsId;
private int quantity;
private double totalPrice;
public Sale(int goodsId, int quantity, double totalPrice) {
this.goodsId = goodsId;
this.quantity = quantity;
this.totalPrice = totalPrice;
}
public int getGoodsId() {
return goodsId;
}
public void setGoodsId(int goodsId) {
this.goodsId = goodsId;
}
public int getQuantity() {
return quantity;
}
public void setQuantity(int quantity) {
this.quantity = quantity;
}
public double getTotalPrice() {
return totalPrice;
}
public void setTotalPrice(double totalPrice) {
this.totalPrice = totalPrice;
}
}
private static class User {
private String username;
private String password;
public User(String username, String password) {
this.username = username;
this.password = password;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
}
```
当然,这只是一个简单的实现,实际开发中需要考虑更多的细节和功能。
相关推荐
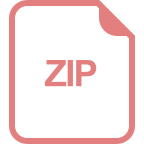
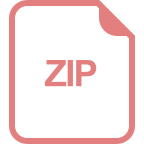
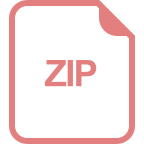














