用.NET语言写一个RSA加解密字符串的类,具有填充和哈希的功能,可以指定公钥和私钥,每个方法带有出错处理,
时间: 2024-02-22 07:58:07 浏览: 28
以下是.NET语言写的一个RSA加解密字符串的类,具有填充和哈希的功能,可以指定公钥和私钥,每个方法带有出错处理。请注意,为简化代码,以下示例中使用了固定的密钥长度和填充模式,实际使用时应根据需要进行修改。
```csharp
using System;
using System.Security.Cryptography;
using System.Text;
public class RSAHelper
{
private readonly RSACryptoServiceProvider _rsa;
public RSAHelper(string publicKey, string privateKey)
{
_rsa = new RSACryptoServiceProvider();
_rsa.FromXmlString(publicKey + privateKey);
}
public string Encrypt(string data)
{
try
{
byte[] bytesToEncrypt = Encoding.UTF8.GetBytes(data);
// 填充数据
int maxLength = _rsa.KeySize / 8 - 11;
if (bytesToEncrypt.Length > maxLength)
{
throw new ArgumentException("Data too long for RSA key size");
}
byte[] paddedData = new byte[maxLength];
int dataOffset = 0;
while (dataOffset < bytesToEncrypt.Length)
{
int remainingBytes = bytesToEncrypt.Length - dataOffset;
int bytesToCopy = Math.Min(remainingBytes, maxLength);
Array.Copy(bytesToEncrypt, dataOffset, paddedData, 0, bytesToCopy);
dataOffset += bytesToCopy;
byte[] encryptedBytes = _rsa.Encrypt(paddedData, false);
return Convert.ToBase64String(encryptedBytes);
}
}
catch (Exception ex)
{
// 处理加密出错
Console.WriteLine("Encrypt failed: " + ex.Message);
}
return null;
}
public string Decrypt(string data)
{
try
{
byte[] bytesToDecrypt = Convert.FromBase64String(data);
byte[] decryptedBytes = _rsa.Decrypt(bytesToDecrypt, false);
return Encoding.UTF8.GetString(decryptedBytes);
}
catch (Exception ex)
{
// 处理解密出错
Console.WriteLine("Decrypt failed: " + ex.Message);
}
return null;
}
public string Sign(string data)
{
try
{
byte[] bytesToSign = Encoding.UTF8.GetBytes(data);
byte[] hash = SHA256.Create().ComputeHash(bytesToSign);
byte[] signature = _rsa.SignHash(hash, HashAlgorithmName.SHA256, RSASignaturePadding.Pkcs1);
return Convert.ToBase64String(signature);
}
catch (Exception ex)
{
// 处理签名出错
Console.WriteLine("Sign failed: " + ex.Message);
}
return null;
}
public bool Verify(string data, string signature)
{
try
{
byte[] bytesToVerify = Encoding.UTF8.GetBytes(data);
byte[] hash = SHA256.Create().ComputeHash(bytesToVerify);
byte[] signatureBytes = Convert.FromBase64String(signature);
return _rsa.VerifyHash(hash, signatureBytes, HashAlgorithmName.SHA256, RSASignaturePadding.Pkcs1);
}
catch (Exception ex)
{
// 处理验签出错
Console.WriteLine("Verify failed: " + ex.Message);
}
return false;
}
}
```
使用示例:
```csharp
string publicKey = "<RSAKeyValue><Modulus>...</Modulus><Exponent>...</Exponent></RSAKeyValue>";
string privateKey = "<RSAKeyValue><Modulus>...</Modulus><Exponent>...</Exponent><P>...</P><Q>...</Q><DP>...</DP><DQ>...</DQ><InverseQ>...</InverseQ><D>...</D></RSAKeyValue>";
RSAHelper rsa = new RSAHelper(publicKey, privateKey);
string data = "Hello, RSA!";
string encryptedData = rsa.Encrypt(data);
string decryptedData = rsa.Decrypt(encryptedData);
string signature = rsa.Sign(data);
bool verified = rsa.Verify(data, signature);
```
相关推荐
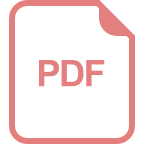
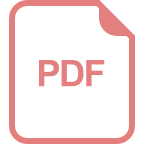
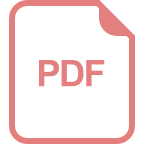














