private static String encrypt(String inputText, String algorithmName) { if (inputText == null || "".equals(inputText.trim())) { throw new IllegalArgumentException("请输入要加密的内容"); } if (algorithmName == null || "".equals(algorithmName.trim())) { algorithmName = "md5"; } String encryptText = null; try { MessageDigest m = MessageDigest.getInstance(algorithmName); m.update(inputText.getBytes("UTF8")); byte[] s = m.digest(); return hex(s); } catch (NoSuchAlgorithmException e) { e.printStackTrace(); } catch (UnsupportedEncodingException e) { e.printStackTrace(); } return encryptText; } /** * 返回十六进制字符串 */ private static String hex(byte[] arr) { StringBuffer sb = new StringBuffer(); for (int i = 0; i < arr.length; ++i) { sb.append(Integer.toHexString((arr[i] & 0xFF) | 0x100) .substring(1, 3)); } return sb.toString(); }使用Python重新写
时间: 2024-01-21 21:03:57 浏览: 67
以下是使用Python重新编写的代码:
```python
import hashlib
def encrypt(inputText, algorithmName="md5"):
if not inputText:
raise ValueError("请输入要加密的内容")
algorithmName = algorithmName.lower()
if algorithmName not in hashlib.algorithms_guaranteed:
raise ValueError("不支持的加密算法")
m = hashlib.new(algorithmName)
m.update(inputText.encode("utf-8"))
s = m.digest()
return hex(s)[2:]
def hex(arr):
return ''.join(format(x, '02x') for x in arr)
```
这是一个更加Pythonic的实现,使用了Python内置的hashlib模块来进行加密。函数的参数和返回值与原代码相同。
相关问题
public String encrypt(String text) throws Exception { if (text == null || text.length() == 0) throw new Exception("Empty string"); byte[] encrypted = null; try { cipher.init(Cipher.ENCRYPT_MODE, keyspec, ivspec); encrypted = cipher.doFinal(padString(text).getBytes()); } catch (Exception e) { throw new Exception("[encrypt] " + e.getMessage()); } return bytesToHex(encrypted); }
这是一个使用Java语言实现的加密函数,它接收一个字符串类型的明文text,返回一个经过加密之后的十六进制字符串。具体的加密算法和密钥长度无法从这个函数中直接确定,需要查看代码中的其他部分或者相关的文档。根据代码中的注释,可以猜测它可能使用了对称加密算法,如AES、DES等,但需要查看代码中的keyspec和ivspec才能确认使用的具体算法和参数。
import javax.swing.*; import java.awt.*; import java.awt.event.*; public class TextEncryptor extends JFrame implements ActionListener { private JLabel label; private JTextField inputText; private JButton encryptButton, decryptButton; private JTextArea outputText; public TextEncryptor() { super("Text Encryptor"); setLayout(new FlowLayout()); label = new JLabel("Enter text:"); add(label); inputText = new JTextField(20); add(inputText); encryptButton = new JButton("Encrypt"); add(encryptButton); encryptButton.addActionListener(this); decryptButton = new JButton("Decrypt"); add(decryptButton); decryptButton.addActionListener(this); outputText = new JTextArea(10, 20); add(outputText); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setSize(300, 300); setVisible(true); } public void actionPerformed(ActionEvent e) { if (e.getSource() == encryptButton) { String input = inputText.getText(); String output = encrypt(input); outputText.setText(output); } else if (e.getSource() == decryptButton) { String input = inputText.getText(); String output = decrypt(input); outputText.setText(output); } } public static void main(String[] args) { new TextEncryptor(); } private String encrypt(String text) { // TODO: 实现加密算法 return text; } private String decrypt(String text) { // TODO: 实现解密算法 return text; } }这段代码使用了什么java基础知识
上述代码运用了以下Java基础知识:
1. GUI编程:使用了Java Swing库创建了一个窗口,其中包括标签、文本框、按钮和文本区域等组件
2. 事件处理:实现了ActionListener接口,对加密和解密按钮的点击事件进行了处理
3. 字符串处理:对用户输入的文本进行字符串的获取和处理,同时实现了加密和解密算法
4. 面向对象编程:使用了面向对象的思想,将加密和解密算法封装在类的私有方法中,提高了程序的可重用性和可维护性
5. 异常处理:未在代码中显式处理异常,但是后续的开发过程中需要注意异常的处理,以保障程序的健壮性。
阅读全文
相关推荐
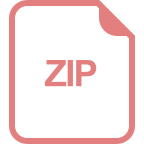
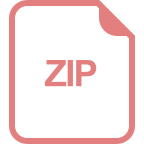
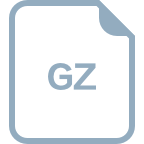
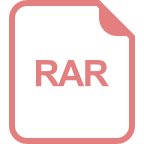
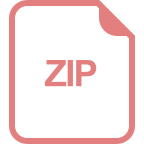
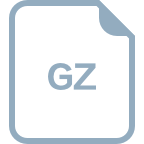
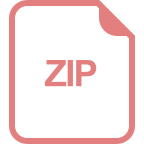
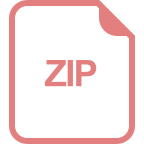
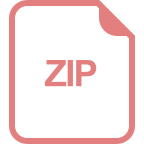
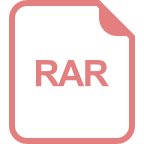
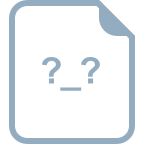

