qtcreator中 一个cpp文件控制另一个文件cpp的线程的恢复和暂停
时间: 2023-12-04 10:01:54 浏览: 106
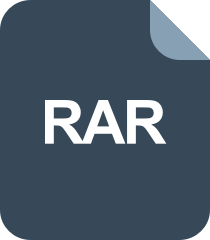
控制线程的启动、暂停、恢复、停止
在 Qt Creator 中,可以使用 Qt 的信号和槽机制来实现一个 CPP 文件控制另一个 CPP 文件的线程恢复和暂停。
假设你有两个 CPP 文件:main.cpp 和 thread.cpp。在 thread.cpp 中,你需要定义一个线程并在其中执行一些操作。在 main.cpp 中,你需要控制该线程的暂停和恢复。
首先,在 thread.cpp 中,你需要定义一个信号 `pauseThread()` 和一个槽函数 `onPauseThread()` 来实现线程的暂停。代码如下:
```cpp
class MyThread : public QThread
{
Q_OBJECT
signals:
void pauseThread();
public slots:
void onPauseThread()
{
// 暂停线程的代码
this->wait();
}
protected:
void run() override
{
// 线程的执行代码
while (true)
{
// ...
QThread::msleep(100);
// ...
}
}
};
```
在 `run()` 函数中,你可以执行一些需要在后台线程中运行的代码。在 `onPauseThread()` 槽函数中,你可以实现暂停线程的代码,例如使用 `wait()` 函数。
然后,在 main.cpp 中,你需要实例化 `MyThread` 类并连接信号和槽函数。代码如下:
```cpp
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
MyThread thread;
thread.start();
QObject::connect(&thread, &MyThread::pauseThread, &thread, &MyThread::onPauseThread);
// ...
return a.exec();
}
```
在 `main()` 函数中,你需要实例化 `MyThread` 类并启动线程。然后,你需要使用 `connect()` 函数将信号 `pauseThread()` 和槽函数 `onPauseThread()` 连接起来。
当你需要暂停线程时,只需要在 main.cpp 中发出 `pauseThread()` 信号即可:
```cpp
// 暂停线程
emit thread.pauseThread();
```
当你需要恢复线程时,只需要在 thread.cpp 中使用 `start()` 函数重新启动线程即可:
```cpp
// 恢复线程
this->start();
```
这样,你就可以在一个 CPP 文件中控制另一个 CPP 文件的线程恢复和暂停了。
阅读全文
相关推荐
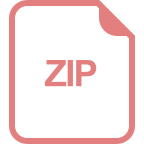
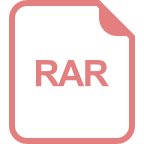
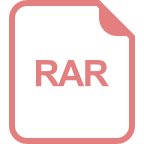
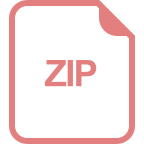
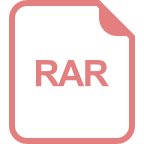
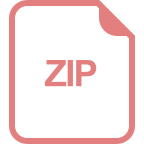
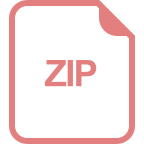
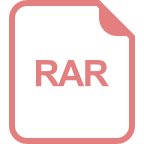
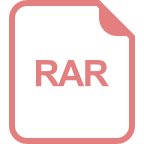
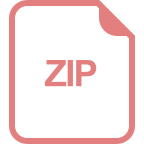
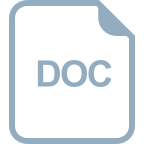
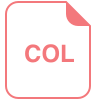
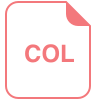
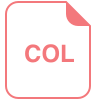
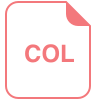
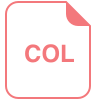
