1、创建file1.PHP文件,设计表单,包含用户名、学号、专业、班级等四方面内容(表单控件形式自拟),点击提交按钮后将以上录入的数据存入cookie 2、创建file2.php,访问页面时,直接获取cookie的内容并显示到网页中
时间: 2024-02-04 20:01:51 浏览: 30
谢谢您向我提问,以下是我的回答:
针对您的问题,我可以为您提供一些思路和代码实现的参考。
1、创建file1.PHP文件,设计表单,包含用户名、学号、专业、班级等四方面内容(表单控件形式自拟),点击提交按钮后将以上录入的数据存入cookie
在file1.PHP文件中,您需要设计一个表单,用于收集用户输入的信息,并在用户点击提交按钮时将收集到的数据存入cookie。
以下是一个示例代码:
```php
<!DOCTYPE html>
<html>
<head>
<title>表单示例</title>
</head>
<body>
<form method="post">
<label for="username">用户名:</label>
<input type="text" id="username" name="username" required><br>
<label for="stuno">学号:</label>
<input type="text" id="stuno" name="stuno" required><br>
<label for="major">专业:</label>
<input type="text" id="major" name="major" required><br>
<label for="class">班级:</label>
<input type="text" id="class" name="class" required><br>
<input type="submit" value="提交">
</form>
<?php
// 判断是否有表单提交
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
// 获取用户输入的数据
$username = $_POST['username'];
$stuno = $_POST['stuno'];
$major = $_POST['major'];
$class = $_POST['class'];
// 将数据存入cookie
setcookie('username', $username, time()+3600);
setcookie('stuno', $stuno, time()+3600);
setcookie('major', $major, time()+3600);
setcookie('class', $class, time()+3600);
// 提示用户数据已保存
echo "<p>数据已保存到cookie中。</p>";
}
?>
</body>
</html>
```
在表单提交后,我们通过$_POST数组获取用户输入的数据,并使用setcookie函数将数据存入cookie中。
2、创建file2.php,访问页面时,直接获取cookie的内容并显示到网页中
在file2.php文件中,我们需要直接获取cookie中存储的数据,并将其显示到网页中。
以下是一个示例代码:
```php
<!DOCTYPE html>
<html>
<head>
<title>显示cookie中的数据</title>
</head>
<body>
<?php
// 判断是否有cookie存在
if (isset($_COOKIE['username'])) {
// 获取cookie中的数据
$username = $_COOKIE['username'];
$stuno = $_COOKIE['stuno'];
$major = $_COOKIE['major'];
$class = $_COOKIE['class'];
// 显示数据
echo "<p>用户名:$username</p>";
echo "<p>学号:$stuno</p>";
echo "<p>专业:$major</p>";
echo "<p>班级:$class</p>";
} else {
echo "<p>没有找到cookie中的数据。</p>";
}
?>
</body>
</html>
```
在file2.php文件中,我们使用isset函数判断是否有cookie存在,如果存在,则通过$_COOKIE数组获取存储在cookie中的数据,并将其显示在网页中。如果不存在,则提示用户没有找到数据。
希望这些代码能够帮助到您。如果您有任何问题,请随时向我提问。
相关推荐
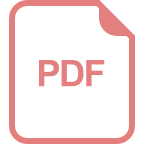
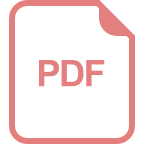
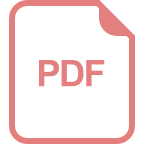














