java如何用刷新界面_java如何按钮刷新
时间: 2023-10-27 19:31:29 浏览: 96
在Java中,可以使用Swing或JavaFX来创建GUI界面。若使用Swing,可以通过以下步骤实现按钮刷新界面:
1. 创建一个JFrame或JPanel来作为界面容器。
2. 在该容器上添加一个JButton。
3. 为按钮添加一个ActionListener,实现按钮点击事件的响应。
4. 在事件响应方法中,可以通过调用repaint()或revalidate()方法来刷新界面。
例如,下面是一个使用Swing实现按钮刷新界面的示例代码:
```
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class RefreshButton extends JFrame {
private JButton refreshButton;
public RefreshButton() {
setTitle("Refresh Button");
setSize(300, 200);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel contentPane = new JPanel(new BorderLayout());
refreshButton = new JButton("Refresh");
refreshButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
// 刷新界面
repaint();
}
});
contentPane.add(refreshButton, BorderLayout.CENTER);
setContentPane(contentPane);
}
public static void main(String[] args) {
RefreshButton frame = new RefreshButton();
frame.setVisible(true);
}
}
```
在上面的代码中,当点击"Refresh"按钮时,会调用repaint()方法来刷新界面。repaint()方法会自动调用paint()方法来重新绘制界面。
如果使用JavaFX来创建GUI界面,可以通过调用setOnAction()方法为按钮添加一个事件处理器,然后在事件处理器中实现刷新界面的逻辑。例如:
```
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class RefreshButton extends Application {
private Button refreshButton;
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Refresh Button");
refreshButton = new Button("Refresh");
refreshButton.setOnAction(e -> {
// 刷新界面
refreshButton.getScene().getWindow().hide();
start(new Stage());
});
StackPane root = new StackPane();
root.getChildren().add(refreshButton);
primaryStage.setScene(new Scene(root, 300, 200));
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
```
在上面的代码中,当点击"Refresh"按钮时,会重新启动应用程序,从而实现刷新界面的效果。
相关推荐
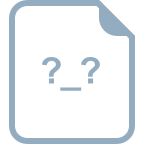
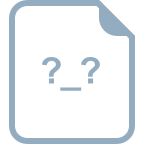
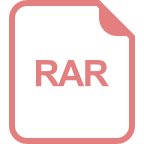














