帮我写一个可以录入试题的题库桌面控制程序代码
时间: 2024-03-22 10:39:52 浏览: 53
好的,为了实现这个功能,我们可以使用Python和Tkinter GUI库来开发题库桌面控制程序。具体的代码如下:
```python
from tkinter import *
import csv
class Question:
def __init__(self, question, option1, option2, option3, option4, answer):
self.question = question
self.option1 = option1
self.option2 = option2
self.option3 = option3
self.option4 = option4
self.answer = answer
class QuestionBank:
def __init__(self):
self.questions = []
def add_question(self, question):
self.questions.append(question)
def save_questions(self):
with open('question_bank.csv', mode='w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Question', 'Option 1', 'Option 2', 'Option 3', 'Option 4', 'Answer'])
for question in self.questions:
writer.writerow([question.question, question.option1, question.option2, question.option3, question.option4, question.answer])
class QuestionBankGUI:
def __init__(self, master):
self.master = master
master.title('Question Bank')
self.question_bank = QuestionBank()
self.question_label = Label(master, text='Question:')
self.question_label.grid(row=0, column=0)
self.question_entry = Entry(master, width=50)
self.question_entry.grid(row=0, column=1, columnspan=3)
self.option1_label = Label(master, text='Option 1:')
self.option1_label.grid(row=1, column=0)
self.option1_entry = Entry(master, width=50)
self.option1_entry.grid(row=1, column=1, columnspan=3)
self.option2_label = Label(master, text='Option 2:')
self.option2_label.grid(row=2, column=0)
self.option2_entry = Entry(master, width=50)
self.option2_entry.grid(row=2, column=1, columnspan=3)
self.option3_label = Label(master, text='Option 3:')
self.option3_label.grid(row=3, column=0)
self.option3_entry = Entry(master, width=50)
self.option3_entry.grid(row=3, column=1, columnspan=3)
self.option4_label = Label(master, text='Option 4:')
self.option4_label.grid(row=4, column=0)
self.option4_entry = Entry(master, width=50)
self.option4_entry.grid(row=4, column=1, columnspan=3)
self.answer_label = Label(master, text='Answer:')
self.answer_label.grid(row=5, column=0)
self.answer_entry = Entry(master, width=50)
self.answer_entry.grid(row=5, column=1, columnspan=3)
self.add_button = Button(master, text='Add Question', command=self.add_question)
self.add_button.grid(row=6, column=0)
self.save_button = Button(master, text='Save Questions', command=self.save_questions)
self.save_button.grid(row=6, column=1)
self.quit_button = Button(master, text='Quit', command=master.quit)
self.quit_button.grid(row=6, column=2)
def add_question(self):
question = Question(self.question_entry.get(), self.option1_entry.get(), self.option2_entry.get(), self.option3_entry.get(), self.option4_entry.get(), self.answer_entry.get())
self.question_bank.add_question(question)
self.question_entry.delete(0, END)
self.option1_entry.delete(0, END)
self.option2_entry.delete(0, END)
self.option3_entry.delete(0, END)
self.option4_entry.delete(0, END)
self.answer_entry.delete(0, END)
def save_questions(self):
self.question_bank.save_questions()
self.master.destroy()
root = Tk()
question_bank_gui = QuestionBankGUI(root)
root.mainloop()
```
这个程序中,我们首先定义了一个Question类,用于存储每个试题的题目、四个选项和正确答案。然后我们又定义了一个QuestionBank类,用于存储所有试题。这个类有两个方法,分别是add_question和save_questions。add_question方法用于向题库中添加一个试题,save_questions方法用于将题库保存到CSV文件中。
最后,我们定义了一个QuestionBankGUI类,用于实现图形用户界面。在这个类中,我们使用了Tkinter库来创建GUI界面,并将所有的试题信息显示在界面上。用户可以通过输入题目、选项和正确答案来添加试题,也可以通过点击"Save Questions"按钮将题库保存到CSV文件中。
如果你想要运行这个程序,只需要将这个程序保存为question_bank.py文件,然后在命令行中运行"python question_bank.py"即可。
阅读全文
相关推荐
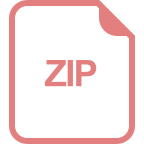
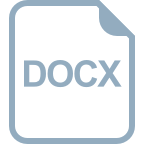
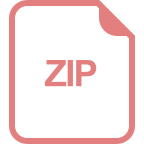
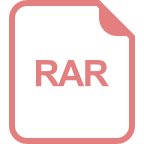
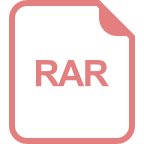
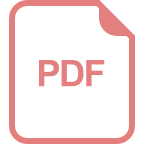
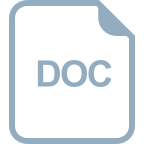
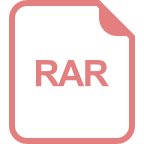
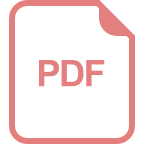
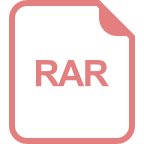
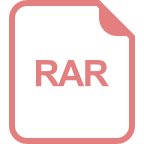
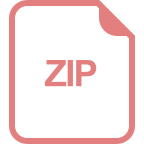
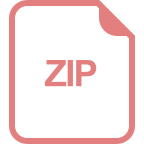
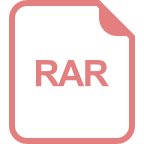
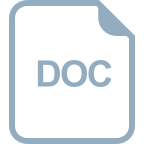
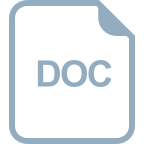