读取Python安装目录中news.txt文件的内容,然后输出其中第100个字符开始的50个字符。
时间: 2024-03-23 22:42:09 浏览: 57
可以使用Python的文件操作和字符串截取来实现这个需求。具体的代码如下:
```python
with open("Python安装目录/news.txt", "r") as f:
content = f.read()
print(content[99:149])
```
其中,`with open("Python安装目录/news.txt", "r") as f:` 打开了名为 `news.txt` 的文件,并将其存储在变量 `f` 中。`"r"` 表示以只读模式打开文件。`content = f.read()` 读取整个文件内容,并将其存储在变量 `content` 中。最后,`print(content[99:149])` 输出第100个字符到第149个字符(共50个字符)的内容。
注意,需要将代码中的 `Python安装目录` 替换为实际的 Python 安装目录。
相关问题
用Python从https://www.chinadaily.com.cn/下载一则新闻,保存到txt文件中,读取该文件,并统计每个单词在新闻中出现的次数。
好的,我可以为您提供帮助。
首先,我们需要使用 Python 的 requests 库来下载指定的新闻网页内容。然后,我们可以使用 BeautifulSoup 库来解析 HTML 页面,并提取出新闻正文的部分。最后,我们可以使用正则表达式或者 Python 内置的字符串处理函数来统计每个单词在新闻中出现的次数。
以下是一个示例代码,您可以在此基础上进行修改和完善:
```python
import requests
from bs4 import BeautifulSoup
import re
# 下载新闻页面的内容
url = "https://www.chinadaily.com.cn/a/202204/29/WS6277fa7ba31099a2340b1c9a.html"
response = requests.get(url)
html = response.text
# 使用 BeautifulSoup 解析 HTML 页面,提取新闻正文
soup = BeautifulSoup(html, "html.parser")
article = soup.find("div", {"class": "article"})
# 提取正文中的文字内容,并去除多余的空格和换行符
text = article.get_text()
text = re.sub(r"\s+", " ", text)
# 将正文内容保存到本地文件中
with open("news.txt", "w", encoding="utf-8") as f:
f.write(text)
# 统计每个单词在新闻中出现的次数
words = text.split()
word_count = {}
for word in words:
if word not in word_count:
word_count[word] = 0
word_count[word] += 1
# 打印每个单词出现的次数
for word, count in word_count.items():
print(word, count)
```
注意:代码中使用的是 Python 3.x 版本。如果您使用的是 Python 2.x 版本,需要对一些函数和语法进行修改。
使用python从https://www.chinadaily.com.cn/下载一则新闻,保存到txt文件中,读取该文件,并统计每个单词在新闻中出现的次数。
好的,我来给您讲一下大致思路。
首先,我们需要使用 Python 的 requests 库来获取网页的 HTML 内容。然后,我们可以使用正则表达式或者 BeautifulSoup 库来提取出新闻的正文部分。接下来,我们可以使用 Python 的文件操作来将新闻保存到 txt 文件中。最后,我们可以使用 Python 的字符串处理函数和数据结构来统计每个单词在新闻中出现的次数。
以下是大致代码:
```python
import requests
from bs4 import BeautifulSoup
# 获取网页内容
url = 'https://www.chinadaily.com.cn/'
response = requests.get(url)
html = response.content
# 提取新闻正文
soup = BeautifulSoup(html, 'html.parser')
news_content = soup.find('div', {'class': 'newsbox'}).text
# 保存到txt文件
with open('news.txt', 'w', encoding='utf-8') as f:
f.write(news_content)
# 统计单词出现次数
word_count = {}
words = news_content.split()
for word in words:
if word not in word_count:
word_count[word] = 1
else:
word_count[word] += 1
# 打印结果
for word, count in word_count.items():
print(word, count)
```
当然,这只是一个简单的示例,实际应用中可能需要针对具体情况进行更多的处理和优化。
阅读全文
相关推荐
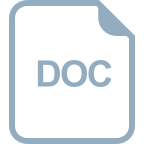
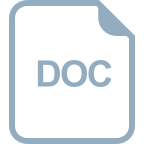
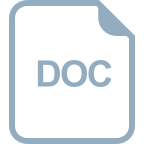
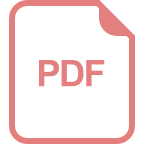
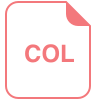
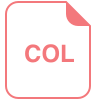

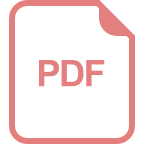
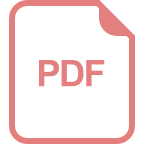
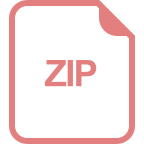
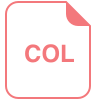
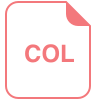
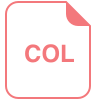
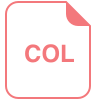
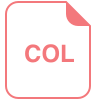
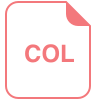
