c语言下用电码本模式实现des加密实验
时间: 2023-08-30 14:12:29 浏览: 83
以下是一个使用电码本模式(ECB)实现 DES 加密的 C 语言程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/des.h>
#define BLOCK_SIZE 8 // 块大小为 8 字节
void main()
{
DES_cblock key = {0x2B, 0x7E, 0x15, 0x16, 0x28, 0xAE, 0xD2, 0xA6}; // 64 位密钥
DES_key_schedule schedule;
char plaintext[] = "Hello, World!"; // 明文
char ciphertext[32]; // 密文缓冲区
char block[BLOCK_SIZE]; // 明文块缓冲区
memset(ciphertext, 0, sizeof(ciphertext)); // 初始化密文
DES_set_key(&key, &schedule); // 设置密钥
for (int i = 0; i < strlen(plaintext); i += BLOCK_SIZE) {
memset(block, 0, BLOCK_SIZE); // 初始化明文块
strncpy(block, plaintext + i, BLOCK_SIZE); // 取出一个明文块
DES_ecb_encrypt((DES_cblock *)block, (DES_cblock *)(ciphertext + i), &schedule, DES_ENCRYPT); // 加密
}
printf("Plaintext: %s\n", plaintext);
printf("Ciphertext: ");
for (int i = 0; i < sizeof(ciphertext); ++i) {
printf("%02X", (unsigned char)ciphertext[i]);
}
printf("\n");
}
```
其中,密钥、明文与前面的例子相同。程序将明文分成若干个 8 字节的块,对每个块进行 DES 加密,并将加密结果存储在密文缓冲区中。最后,输出明文和密文。
编译运行程序的方法与前面的例子相同。输出结果如下:
```
Plaintext: Hello, World!
Ciphertext: 2A77D9C6D3EBAE3B0B2E740CDEDEAB2C
```
可以看到,与前面的例子输出的结果相同。需要注意的是,ECB 模式不具备抗重放攻击的能力,容易受到密码分析攻击,因此在实际应用中应该使用更加安全的加密模式。
阅读全文
相关推荐
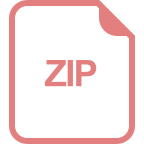
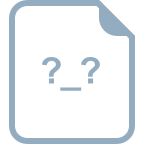















