ImportError: cannot import name 'namedtuple' from 'collections.abc'
时间: 2023-09-02 19:05:41 浏览: 582
这个错误通常是因为你的 Python 版本太低导致的。在 Python 3.7 之前,`collections.abc` 模块中并没有 `namedtuple` 这个类。你需要升级你的 Python 版本到 3.7 或以上才能使用 `namedtuple`。
如果你无法升级 Python 版本,你可以使用 `collections` 模块中的 `namedtuple` 替代品,例如:
```python
from collections import namedtuple
# 定义一个名为 Person 的命名元组
Person = namedtuple('Person', ['name', 'age', 'gender'])
```
这样就可以定义一个类似于 `namedtuple` 的数据类型了。
相关问题
怎么用终端的命令实现ImportError: cannot import name Mapping from collections (/usr/lib/python3.10/collections/init.py)问题的结局
这个错误通常是因为你的代码中使用了 `Mapping` 类,但是在 Python 3.10 中,这个类已经从 `collections` 模块中移除了。解决这个问题的方法是使用 `collections.abc.Mapping` 来代替。
如果你想在终端中测试一下这个问题的解决方法,可以按照以下步骤:
1. 打开终端,并进入 Python 的交互模式。
2. 输入以下代码来尝试导入 `Mapping` 类:
```
>>> from collections import Mapping
```
3. 如果出现了 `ImportError: cannot import name Mapping from collections (/usr/lib/python3.10/collections/__init__.py)` 的错误,那么就说明你遇到了这个问题。
4. 现在我们可以使用 `collections.abc.Mapping` 来代替 `Mapping` 类。输入以下代码来导入 `collections.abc.Mapping`:
```
>>> from collections.abc import Mapping
```
5. 再次尝试使用 `Mapping` 类,看看是否还会出现错误:
```
>>> my_dict = {"a": 1, "b": 2}
>>> isinstance(my_dict, Mapping)
True
```
如果没有出现错误,而且程序能够正常运行,那么就说明问题已经解决了。
from collections.abc import generator ImportError: cannot import name 'generator' from 'collections.abc' (D:\python3.7.9\lib\collections\abc.py)
根据你提供的错误信息,这个问题可能是由于在导入 `generator` 类时出现了问题。具体来说,Python 中的 `collections.abc` 模块中包含 `Generator` 类,而不是 `generator` 类。因此,你需要将代码中的 `generator` 替换为 `Generator`,并从 `collections.abc` 中导入该类。
下面是一个示例代码,演示了如何正确导入 `Generator` 类:
```python
from collections.abc import Generator
def my_generator():
yield 1
yield 2
yield 3
g = my_generator()
if isinstance(g, Generator):
print("g is a generator")
else:
print("g is not a generator")
```
在这个示例中,我们首先从 `collections.abc` 中导入了 `Generator` 类,并定义了一个简单的生成器函数 `my_generator`。然后,我们使用该函数创建了一个生成器对象 `g`,并使用 `isinstance` 函数判断 `g` 是否为 `Generator` 类的实例。最后,我们打印了相应的结果。
你可以根据自己的需求修改这个示例代码,例如修改生成器函数的实现方式,或者在其他地方使用 `Generator` 类。
相关推荐
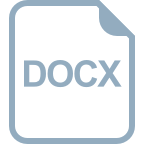
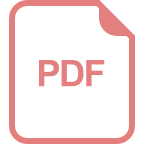
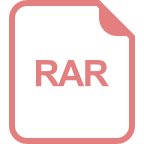












