c语言实现字符串替换字符串
时间: 2023-09-15 19:17:57 浏览: 1970
可以使用C语言中提供的字符串函数`strstr()`和`strcpy()`来实现字符串替换字符串的功能。具体步骤如下:
1. 在源字符串中查找需要替换的子串的位置,可以使用`strstr()`函数;
2. 如果查找到了子串,就先将源字符串中子串后面的所有字符往后移动,然后再将新的字符串插入到子串的位置上,可以使用`strcpy()`函数;
3. 重复上述步骤,直到源字符串中不再含有需要替换的子串。
下面是一个示例代码,用于将字符串中的所有`old_str`替换为`new_str`:
```c
#include <stdio.h>
#include <string.h>
void replace_str(char *str, const char *old_str, const char *new_str) {
int old_len = strlen(old_str);
int new_len = strlen(new_str);
char *pos = str;
while ((pos = strstr(pos, old_str)) != NULL) {
// 将源字符串中子串后面的所有字符往后移动
memmove(pos + new_len, pos + old_len, strlen(pos + old_len) + 1);
// 将新的字符串插入到子串的位置上
memcpy(pos, new_str, new_len);
pos += new_len;
}
}
int main() {
char str[100] = "hello world, hello C!";
replace_str(str, "hello", "hi");
printf("%s\n", str);
return 0;
}
```
输出结果为:`hi world, hi C!`
阅读全文
相关推荐
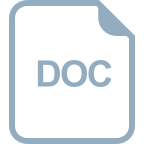
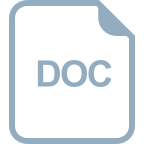
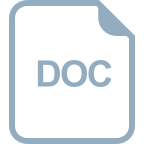
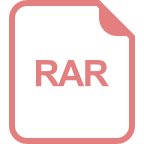
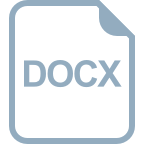
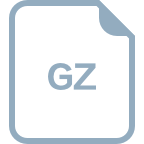
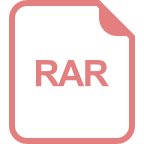
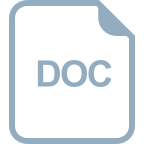
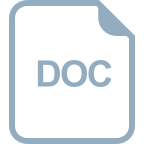
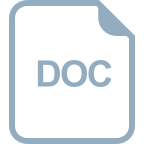
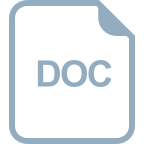


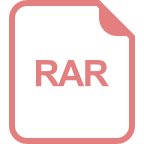
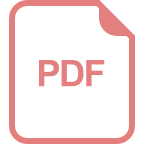
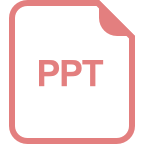
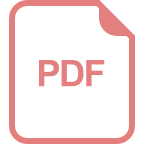
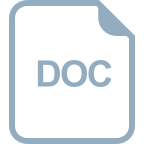