nestjs,监听真实区块链数据上各种代币的最新价格,websocket ,写代码
时间: 2024-06-10 13:09:59 浏览: 15
可以使用NestJS框架的WebSocket模块和第三方的区块链API来实现监听真实区块链数据上各种代币的最新价格。下面是一个简单的实现示例:
1. 安装WebSocket模块和区块链API
```bash
npm install @nestjs/websockets --save
npm install web3 --save
```
2. 创建WebSocket服务
```typescript
import { WebSocketGateway, WebSocketServer } from '@nestjs/websockets';
import { Server } from 'socket.io';
@WebSocketGateway()
export class PriceGateway {
@WebSocketServer() server: Server;
constructor() {
// 连接区块链API
const web3 = new Web3('https://mainnet.infura.io/v3/your-api-key');
// 监听代币价格变化
const tokenContract = new web3.eth.Contract(tokenABI, tokenAddress);
tokenContract.events.Transfer({}).on('data', event => {
const { returnValues: { from, to, value } } = event;
// 计算最新价格
const price = value / 10 ** decimals;
// 发送价格到WebSocket客户端
this.server.emit('price', { from, to, price });
});
}
}
```
3. 注册WebSocket服务
```typescript
import { Module } from '@nestjs/common';
import { PriceGateway } from './price.gateway';
@Module({
providers: [PriceGateway],
})
export class PriceModule {}
```
4. 启动WebSocket服务
```typescript
import { NestFactory } from '@nestjs/core';
import { AppModule } from './app.module';
async function bootstrap() {
const app = await NestFactory.create(AppModule);
app.listen(3000, () => console.log('Server is running on http://localhost:3000'));
}
bootstrap();
```
5. 创建WebSocket客户端
```typescript
const socket = io('http://localhost:3000');
socket.on('price', data => {
console.log('New price:', data);
});
```
注意:以上代码是示例代码,需要根据具体的代币和区块链API进行调整。另外,这种方式只能获取到已经发生的价格变化,不能保证实时性。如果需要实时获取价格变化,可以考虑使用其他方式,如轮询或订阅区块链API提供的WebSocket接口。
相关推荐
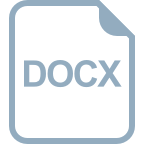
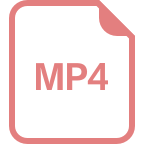














