bool Insert( List L, ElementType X ){ if(L->Last+1>=MAXSIZE) return false; for (int i=0; i<=L->Last; i++) { if(X == L->Data[i]) return false; if(X>L->Data[i]) { for (int j=L->Last+1; j>i; j--) L->Data[j]=L->Data[j-1]; L->Data[i]=X; L->Last++; return true; } if(i==L->Last&&L->Data[i]>X) { L->Data[L->Last+1]=X; L->Last++; return true; } } }补全代码
时间: 2024-03-25 13:39:32 浏览: 21
需要补全的代码如下:
```c++
#define MAXSIZE 100 /* 链表的最大长度 */
typedef int ElementType;
typedef struct LNode *List;
struct LNode {
ElementType Data[MAXSIZE];
int Last;
};
bool Insert(List L, ElementType X) {
if (L->Last + 1 >= MAXSIZE)
return false;
for (int i = 0; i <= L->Last; i++) {
if (X == L->Data[i])
return false;
if (X > L->Data[i]) {
for (int j = L->Last + 1; j > i; j--)
L->Data[j] = L->Data[j-1];
L->Data[i] = X;
L->Last++;
return true;
}
if (i == L->Last && L->Data[i] > X) {
L->Data[L->Last + 1] = X;
L->Last++;
return true;
}
}
return false; /* 如果插入失败,需要返回 false */
}
```
相关推荐
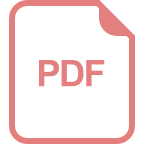
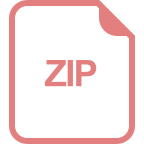















