springcloud注入bean的类里面查询数据库
时间: 2023-08-25 19:39:00 浏览: 98
要在Spring Cloud应用程序中查询数据库并注入到Bean中,您可以使用Spring Data JPA或MyBatis等持久层框架。
在使用Spring Data JPA的情况下,您可以使用@Repository注解在应用程序中定义存储库类,并使用@Autowired注解将其注入到您的服务中。以下是一个简单的示例:
```
@Repository
public class UserRepositoryImpl implements UserRepositoryCustom {
@PersistenceContext
private EntityManager entityManager;
@Override
public List<User> findUsersByAgeGreaterThan(int age) {
CriteriaBuilder cb = entityManager.getCriteriaBuilder();
CriteriaQuery<User> query = cb.createQuery(User.class);
Root<User> root = query.from(User.class);
query.select(root).where(cb.greaterThan(root.get("age"), age));
return entityManager.createQuery(query).getResultList();
}
}
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserRepositoryCustom userRepositoryCustom;
@Override
public List<User> getUsersByAgeGreaterThan(int age) {
return userRepositoryCustom.findUsersByAgeGreaterThan(age);
}
}
```
在上面的代码中,@Repository注解用于定义一个Spring Data JPA存储库类。在存储库类中,您可以使用@PersistenceContext注解注入EntityManager实例,并使用Criteria API构建查询语句。在服务类中,您可以使用@Autowired注解将存储库类注入到服务中,并调用自定义查询方法。
在使用MyBatis的情况下,您需要在应用程序中定义一个Mapper接口,并在应用程序中创建一个SqlSessionFactory实例。然后,您可以使用@Autowired注解将Mapper接口注入到您的服务中,并调用Mapper接口中定义的方法。以下是一个简单的示例:
```
@Mapper
public interface UserMapper {
@Select("SELECT * FROM user WHERE age > #{age}")
List<User> findUsersByAgeGreaterThan(@Param("age") int age);
}
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Override
public List<User> getUsersByAgeGreaterThan(int age) {
return userMapper.findUsersByAgeGreaterThan(age);
}
}
```
在上面的代码中,@Mapper注解用于定义一个Mapper接口。在Mapper接口中,您可以使用@Select注解定义从数据库中检索数据的SQL查询语句。在服务类中,您可以使用@Autowired注解将Mapper接口注入到服务中,并调用Mapper接口中定义的方法。
无论使用哪种持久层框架,您需要在应用程序中配置数据源,并将其与持久层框架关联起来。例如,在使用Spring Data JPA的情况下,您可以在application.properties文件中添加以下配置项:
```
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=root
spring.datasource.password=admin
spring.datasource.driverClassName=com.mysql.cj.jdbc.Driver
spring.jpa.database-platform=org.hibernate.dialect.MySQLDialect
spring.jpa.show-sql=true
spring.jpa.hibernate.ddl-auto=update
```
在上面的代码中,您需要将URL、用户名和密码设置为您的MySQL数据库连接信息,并将驱动程序类名设置为com.mysql.cj.jdbc.Driver。此外,您还需要设置Hibernate方言、是否显示SQL语句和自动DDL生成策略。
阅读全文
相关推荐

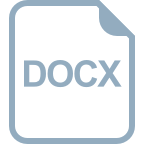
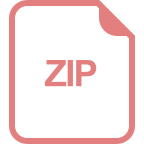
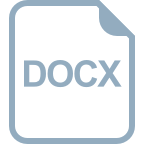
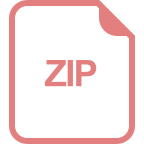
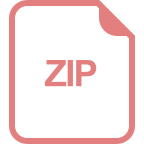
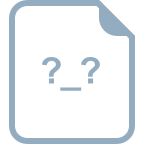
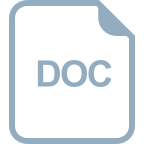
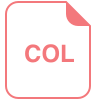
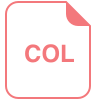
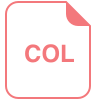
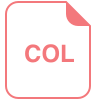
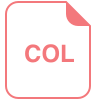
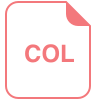
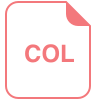
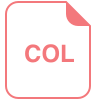