mypaties-plus多对多SQL在mapper
时间: 2024-02-23 19:01:38 浏览: 131
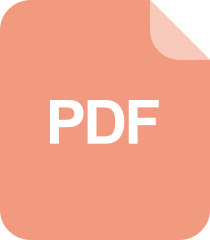
MyBatis-Plus 分页查询以及自定义sql分页的实现

MyBatis-Plus提供了方便的多对多SQL语句操作方法,可以使用`@ManyToMany`注解来配置多对多关系,不需要手动编写SQL语句。
假设有两个表:Student(学生)和 Course(课程),一个学生可以选修多个课程,一个课程可以被多个学生选修。在 Student 表中,有一个 studentId 字段作为主键,在 Course 表中,有一个 courseId 字段作为主键。两个表之间使用一个中间表 StudentCourse(学生课程关系表)来维护多对多关系,其中有一个 studentId 字段和一个 courseId 字段。
在 Student 实体类中,定义如下字段和注解:
```java
public class Student {
@TableId(type = IdType.AUTO)
private Long id;
private String name;
@TableField(exist = false)
private List<Course> courses;
// getter 和 setter 方法
}
```
在 Course 实体类中,定义如下字段和注解:
```java
public class Course {
@TableId(type = IdType.AUTO)
private Long id;
private String name;
@TableField(exist = false)
private List<Student> students;
// getter 和 setter 方法
}
```
在 StudentMapper 接口中,定义如下方法:
```java
public interface StudentMapper extends BaseMapper<Student> {
@Select("SELECT c.* FROM course c INNER JOIN student_course sc ON c.id = sc.course_id WHERE sc.student_id = #{studentId}")
List<Course> getCoursesByStudentId(Long studentId);
}
```
在 CourseMapper 接口中,定义如下方法:
```java
public interface CourseMapper extends BaseMapper<Course> {
@Select("SELECT s.* FROM student s INNER JOIN student_course sc ON s.id = sc.student_id WHERE sc.course_id = #{courseId}")
List<Student> getStudentsByCourseId(Long courseId);
}
```
这样,就可以在 Java 代码中方便地使用 MyBatis-Plus 来处理多对多关系了。如果需要插入、删除中间表的记录,可以使用 MyBatis-Plus 提供的`insertBatchSomeColumn`和`deleteBatchByIds`方法。
阅读全文
相关推荐
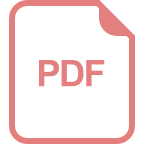
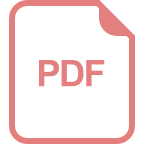
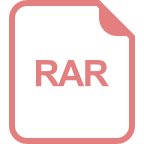
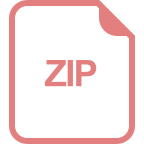
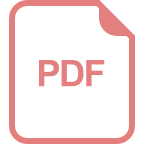
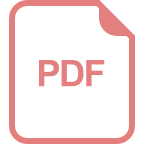
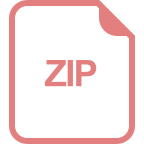
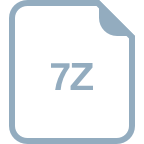
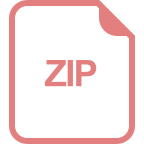
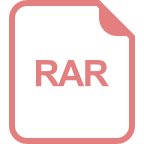