字符串查找 c代码
时间: 2023-07-15 22:15:42 浏览: 54
以下是一个简单的字符串查找函数的C代码示例:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello, world!";
char *ptr = strstr(str, "world");
if (ptr != NULL) {
printf("'%s' was found in '%s'\n", ptr, str);
}
else {
printf("'%s' was not found in '%s'\n", "world", str);
}
return 0;
}
```
这个程序使用了C语言标准库中的 `strstr()` 函数来查找字符串中是否包含另一个字符串。该函数返回一个指向第一个匹配子串的指针,或者返回 `NULL` 如果未找到匹配的子串。在上面的例子中,我们使用 `strstr()` 函数来查找 "world" 子串是否在 "Hello, world!" 字符串中出现。如果找到了匹配子串,则程序会打印一条消息表示已找到,否则打印另一条消息表示未找到。
相关问题
c语言单个字符串查找字符
在C语言中,可以使用strchr函数来查找单个字符在字符串中的位置。该函数原型如下:
```c
char *strchr(const char *str, int c);
```
其中,`str`表示要查找的字符串,`c`表示要查找的字符。函数返回值为指向要查找字符位置的指针,如果找不到则返回NULL。
下面是一个示例代码,演示如何使用strchr函数查找单个字符在字符串中的位置:
```c
#include <stdio.h>
#include <string.h>
int main()
{
char str[] = "hello";
char c = 'e';
char *p = strchr(str, c);
if (p != NULL) {
printf("Found %c at position %ld\n", c, p - str);
} else {
printf("Not found\n");
}
return 0;
}
```
输出结果为:
```
Found e at position 1
```
可以看到,代码中使用`strchr`函数查找字符`'e'`在字符串`"hello"`中的位置,并输出了结果。
C语言二维数组实现字符串查找
以下是C语言二维数组实现字符串查找的代码示例:
```c
#include <stdio.h>
#include <string.h>
int main() {
int N;
while(scanf("%d", &N), N != 0) {
char s[100][100];
int i;
int max_i, max_len = 0;
for(i = 0; i < N; i ++) {
scanf("%s", s[i]);
}
for(i = 0; i < N; i ++) {
int l = strlen(s[i]);
if(max_len < l) {
max_len = l;
max_i = i;
}
}
printf("%s\n", s[max_i]);
}
return 0;
}
```
该代码使用二维字符数组s来存储输入的字符串,通过循环遍历数组中的每个字符串,使用strlen函数获取字符串长度,然后比较长度大小,找到最长的字符串并输出。
阅读全文
相关推荐
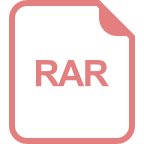
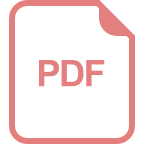
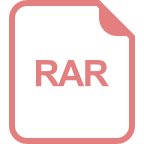
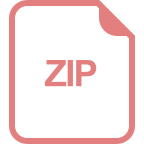
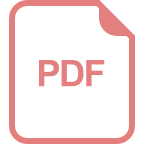
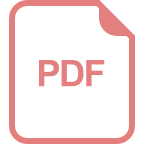
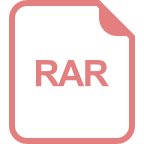
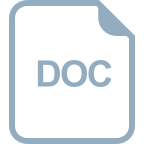
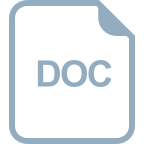




