c++容器实现设计一个员工类 计算员工的薪资 公司有5个员工 按照员工工资排序,输出员工的工号
时间: 2024-01-22 20:17:17 浏览: 26
下面是一个使用 vector 容器实现的员工类和排序方法的示例代码:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
class Employee {
public:
Employee(int id, double salary) : id(id), salary(salary) {}
int getId() const { return id; }
double getSalary() const { return salary; }
private:
int id;
double salary;
};
bool compareBySalary(const Employee& e1, const Employee& e2) {
return e1.getSalary() > e2.getSalary();
}
int main() {
vector<Employee> employees = {
Employee(1, 5000),
Employee(2, 8000),
Employee(3, 6000),
Employee(4, 7500),
Employee(5, 4500)
};
sort(employees.begin(), employees.end(), compareBySalary);
cout << "按照员工工资排序,输出员工的工号:" << endl;
for (const auto& employee : employees) {
cout << employee.getId() << endl;
}
return 0;
}
```
在上面的代码中,Employee 类有两个成员变量:id 和 salary,分别表示员工的工号和薪资。compareBySalary 函数是一个比较函数,用于按照员工工资进行排序。在 main 函数中,我们使用 vector 容器来存储 5 个员工对象,并按照员工工资进行排序,最后输出员工的工号。
相关推荐
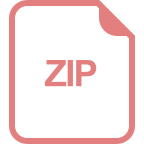
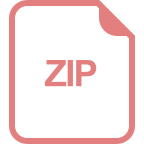
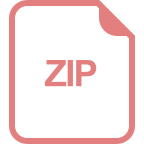














