python将uint字段转化为string
时间: 2024-02-13 18:01:32 浏览: 270
在 Python 中,可以使用 `str()` 函数将一个 `uint` 类型的整数转换为字符串类型。
示例代码:
```python
uint_num = 123
str_num = str(uint_num)
print(type(str_num)) # 输出结果为 <class 'str'>
```
输出:
```
<class 'str'>
```
另外,如果您需要在转换时保留前导零,可以使用 `zfill()` 函数进行填充。
示例代码:
```python
uint_num = 7
str_num = str(uint_num).zfill(3)
print(str_num) # 输出结果为 '007'
```
输出:
```
007
```
相关问题
验证码库python
### Python验证码库推荐
#### 使用Pillow处理图像验证码
对于简单的图形变换和基础的图像操作,`Pillow`是一个非常实用的选择。该库提供了读取、保存多种图片文件格式的功能,并支持基本的几何转换和其他常见的图像处理功能[^1]。
```python
from PIL import Image, ImageDraw, ImageFont
def create_captcha(text="ABCD", width=200, height=80):
image = Image.new('RGB', (width, height), color=(255, 255, 255))
font = ImageFont.truetype("arial.ttf", size=40)
draw = ImageDraw.Draw(image)
w, h = draw.textsize(text, font=font)
draw.text(((width-w)/2,(height-h)/2), text, fill="black", font=font)
return image
```
#### 利用OpenCV进行复杂图像预处理
当面对更复杂的场景时,比如扭曲变形的文字或带有干扰线的情况,则可能需要用到更为强大的工具——`OpenCV`来进行前期清理工作,提高后续识别的成功率。
```python
import cv2
import numpy as np
def preprocess_image(image_path='captcha.png'):
img_gray = cv2.imread(image_path, cv2.IMREAD_GRAYSCALE)
_, thresh_img = cv2.threshold(img_gray, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)
kernel = np.ones((3, 3), np.uint8)
opening = cv2.morphologyEx(thresh_img, cv2.MORPH_OPEN, kernel, iterations=2)
sure_bg = cv2.dilate(opening, kernel, iterations=3)
dist_transform = cv2.distanceTransform(opening, cv2.DIST_L2, 5)
_, sure_fg = cv2.threshold(dist_transform, 0.7 * dist_transform.max(), 255, 0)
sure_fg = np.uint8(sure_fg)
unknown = cv2.subtract(sure_bg, sure_fg)
contours, _ = cv2.findContours(sure_fg.copy(), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)[-2:]
mask = np.zeros_like(img_gray)
for cnt in contours:
x,y,w,h = cv2.boundingRect(cnt)
if min(w,h)>10: # filter out small regions which are likely noise
cv2.rectangle(mask,(x,y),(x+w,y+h),255,-1)
result = cv2.bitwise_and(img_gray,img_gray,mask=mask)
return result
```
#### 应用PyTesseract实现OCR文字识别
如果目标是从经过适当处理后的图像中提取文本信息,那么可以借助于基于Google Tesseract引擎构建而成的`pytesseract`来完成这项任务。
```python
import pytesseract
def recognize_text_from_image(image_path='processed_captcha.png'):
custom_config = r'--oem 3 --psm 6'
recognized_text = pytesseract.image_to_string(Image.open(image_path), config=custom_config)
return recognized_text.strip()
```
#### Django Simple Captcha集成至Web应用
针对希望快速搭建具备验证码验证能力web应用程序的需求者来说,`django-simple-captcha`提供了一种便捷的方式,在Django项目里轻松加入这一特性[^3]。
```bash
pip install django-simple-captcha
```
在settings.py添加配置:
```python
INSTALLED_APPS += ['captcha']
```
并在urls.py注册路由:
```python
urlpatterns += [
path('captcha/', include('captcha.urls')),
]
```
最后可以在表单类Form中使用CaptchaField字段即可。
#### DDDDocr高效准确地解析各类验证码
作为一款专注于中文环境下的验证码解决方案,`ddddocr`以其简单易用性和较高的准确性著称,尤其适合那些追求效率而不愿意花费过多时间调整参数的应用场合[^4]。
```python
import ddddocr
def decode_with_ddddocr(image_file='code.png'):
ocr = ddddocr.DdddOcr()
with open(image_file, 'rb') as f:
img_bytes = f.read()
prediction = ocr.classification(img_bytes)
return prediction
```
通过上述介绍可以看出,不同类型的Python验证码库各有侧重,开发者可以根据具体应用场景和个人偏好做出合适的选择。
python用ctypes的structure解析数据包
在Python中,`ctypes`模块主要用于访问操作系统提供的C语言动态链接库(DLL),同时也支持结构体(Structure)的创建和解析。当你需要处理包含复杂数据类型的网络数据包,比如二进制协议数据,可以使用`ctypes.Structure`来定义一个结构体,这个结构体描述了数据包的数据布局。
首先,你需要创建一个`ctypes.Structure`类,给每个字段指定名字、类型和长度(如果需要)。例如:
```python
import ctypes
class PacketData(ctypes.Structure):
_fields_ = [
('header', ctypes.c_uint32), # 整数,32位
('payload', ctypes.c_char * 1024) # 字符串,最多1024字节
]
```
然后,你可以使用`ctypes.create_string_buffer`来从接收的数据流创建一个缓冲区,接着通过`cast()`函数将缓冲区转换成对应的结构体类型:
```python
data_buf = bytes.fromhex('your_data_hex') # 示例:假设收到的数据是十六进制字符串
buffer = ctypes.create_string_buffer(data_buf)
packet = PacketData.from_address(buffer.raw) # 解析数据包到PacketData实例
```
现在,你可以像操作普通的Python对象一样访问结构体的成员:
```python
header = packet.header
payload = packet.payload.decode() # 如果payload是二进制字符串
```
阅读全文
相关推荐



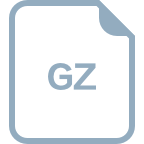











