构造函数必须是公有属性吗
时间: 2024-01-26 16:26:25 浏览: 24
构造函数可以是公有属性、保护属性、私有属性,但是它们的访问权限会影响到对象的创建和初始化。
如果构造函数是公有属性,那么任何地方都可以创建对象,并且可以在类的外部或派生类中调用构造函数进行对象的初始化。这是最常见的构造函数访问权限,并且也是最常用的。
如果构造函数是保护属性或私有属性,那么只有类的内部或派生类可以调用构造函数进行对象的初始化。这种情况通常用于实现单例模式或工厂模式等特殊的设计模式。
总之,构造函数的访问权限应该根据具体的需求来确定,以保证对象的创建和初始化能够正确地进行。
相关问题
python构造日期类,包括年、月、日等私有属性,构造函数和操作这些私有属性的公有函
下面是一个Python构造日期类的示例代码,包括年、月、日等私有属性、构造函数和操作这些私有属性的公有方法:
```python
class Date:
def __init__(self, year, month, day):
self.__year = year
self.__month = month
self.__day = day
def get_year(self):
return self.__year
def set_year(self, year):
self.__year = year
def get_month(self):
return self.__month
def set_month(self, month):
self.__month = month
def get_day(self):
return self.__day
def set_day(self, day):
self.__day = day
```
在这个类中,我们使用双下划线将年、月、日属性定义为私有属性,这样它们就只能在类的内部访问。构造函数`__init__()`用于初始化这些私有属性。我们还定义了一些公有方法用于获取和设置这些私有属性,这样我们就可以在类的外部访问和修改它们。
例如,如果我们要创建一个名为`d`的日期对象,并将其年份设置为2021年,我们可以这样做:
```python
d = Date(2021, 1, 1)
d.set_year(2021)
```
注意,我们不能直接访问`d.__year`,因为它是私有属性。相反,我们必须使用公有方法`get_year()`和`set_year()`来访问和修改它。
构造日期类,包括年、月、日等私有属性,构造函数和操作这些私有属性的公有函数以及将该对象转换成字符串的转换函数(就是能强制类型转换成string类,比如:有一个日期对象d=date(2019,3,26), 则string(d)就会返回一个字符串“2019年3月26日”);构造人员类,包括姓名,出生日期(复合了日期类的对象)等私有属性,构造函数和操作这些属性的公有函数;构造学生类,该类从人员类派生而来,包括学号和专业私有属性,构造函数和操作私有属性的公有函数。
好的,这是一个面向对象编程的问题,我可以帮您给出一种可能的实现方式,代码如下:
```c++
#include <iostream>
#include <string>
#include <sstream>
using namespace std;
class Date {
private:
int year;
int month;
int day;
public:
Date(int y, int m, int d) {
year = y;
month = m;
day = d;
}
void setYear(int y) {
year = y;
}
void setMonth(int m) {
month = m;
}
void setDay(int d) {
day = d;
}
int getYear() const {
return year;
}
int getMonth() const {
return month;
}
int getDay() const {
return day;
}
string toString() {
stringstream ss;
ss << year << "年" << month << "月" << day << "日";
return ss.str();
}
};
class Person {
private:
string name;
Date birth;
public:
Person(string n, Date b) {
name = n;
birth = b;
}
void setName(string n) {
name = n;
}
void setBirth(Date b) {
birth = b;
}
string getName() const {
return name;
}
Date getBirth() const {
return birth;
}
};
class Student : public Person {
private:
string id;
string major;
public:
Student(string n, Date b, string i, string m) : Person(n, b) {
id = i;
major = m;
}
void setId(string i) {
id = i;
}
void setMajor(string m) {
major = m;
}
string getId() const {
return id;
}
string getMajor() const {
return major;
}
};
int main() {
Date d(2019, 3, 26);
cout << string(d) << endl;
Person p("张三", d);
cout << p.getName() << "的生日是" << string(p.getBirth()) << endl;
Student s("李四", d, "201901", "计算机科学与技术");
cout << s.getName() << "的生日是" << string(s.getBirth()) << ",学号是" << s.getId() << ",专业是" << s.getMajor() << endl;
return 0;
}
```
这里定义了三个类,分别是日期类 `Date`、人员类 `Person` 和学生类 `Student`。其中,人员类和学生类都是从日期类派生而来的,因为它们都需要包含生日这个属性。
日期类包含年、月、日三个私有属性,以及构造函数和操作这些属性的公有函数,还包含将日期对象转换为字符串的函数 `toString()`。
人员类包含姓名和生日两个私有属性,以及构造函数和操作这些属性的公有函数。
学生类包含学号和专业两个私有属性,以及构造函数和操作这些属性的公有函数。注意,学生类的构造函数要调用父类 `Person` 的构造函数来初始化姓名和生日属性。
在 `main()` 函数中,我们创建了一个日期对象 `d`,创建了一个人员对象 `p`,以及创建了一个学生对象 `s`。我们分别调用了它们的属性和方法,并将日期对象和人员对象转换为字符串。可以看到,转换为字符串的格式符合题目要求。
相关推荐
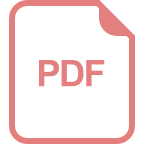
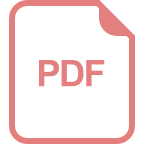
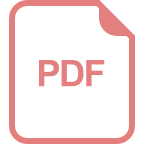











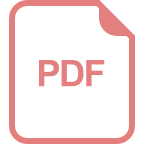
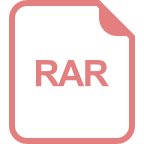