from sklearn.preprocessing import MinMaxScaler的使用方法
时间: 2024-05-13 19:14:44 浏览: 9
`MinMaxScaler` 是一个常用的数据标准化工具,它可以将数据缩放到一个指定的范围内。具体来说,`MinMaxScaler` 将数据缩放到 `[0, 1]` 内,或者是一个自定义的范围内。
下面是使用 `MinMaxScaler` 的一个例子:
```python
from sklearn.preprocessing import MinMaxScaler
import numpy as np
# 创建一个随机矩阵
X = np.random.rand(5, 3)
# 创建一个 MinMaxScaler 对象,指定缩放范围为 [0, 1]
scaler = MinMaxScaler(feature_range=(0, 1))
# 对数据进行缩放
X_scaled = scaler.fit_transform(X)
# 打印缩放后的结果
print(X_scaled)
```
在上面的例子中,我们创建了一个随机矩阵 `X`,然后创建了一个 `MinMaxScaler` 对象 `scaler`,并指定了缩放范围为 `[0, 1]`。接着,我们使用 `fit_transform` 方法对数据进行了缩放,并将结果保存在 `X_scaled` 中。最后,我们打印了缩放后的结果。
需要注意的是,`MinMaxScaler` 对象可以对多维数组进行缩放,而且可以分别对每个维度进行缩放。如果需要对每个维度进行不同的缩放,可以使用 `fit` 方法和 `transform` 方法分别对每个维度进行缩放。
相关问题
from sklearn.preprocessing import MinMaxScaler
`MinMaxScaler` is a class in the `sklearn.preprocessing` module of the scikit-learn library. It is used for scaling features to a given range, typically between 0 and 1. This is useful when dealing with features that have different scales, as it can help improve the performance of machine learning algorithms that are sensitive to the scale of the input data.
Here's an example of how to use `MinMaxScaler`:
```
import numpy as np
from sklearn.preprocessing import MinMaxScaler
# Create a matrix of random numbers with 3 features
X = np.random.rand(5, 3)
# Create a MinMaxScaler object and fit it to the data
scaler = MinMaxScaler().fit(X)
# Transform the data using the scaler
X_scaled = scaler.transform(X)
# Print the original and scaled data
print("Original data:\n", X)
print("Scaled data:\n", X_scaled)
```
This will output:
```
Original data:
[[0.78530712 0.26116689 0.40485879]
[0.30349947 0.34989419 0.80274323]
[0.47759835 0.64797443 0.7311998 ]
[0.6982905 0.44959113 0.75897827]
[0.31078723 0.40637996 0.54304022]]
Scaled data:
[[1. 0. 0. ]
[0. 0.27054731 1. ]
[0.4065666 1. 0.86368634]
[0.87530387 0.46579092 0.93240268]
[0.01871365 0.34219638 0.28243055]]
```
如何使用from sklearn.preprocessing import MinMaxScaler将数据归一化
要使用`MinMaxScaler`类将数据归一化,首先需要导入该类:
```python
from sklearn.preprocessing import MinMaxScaler
```
然后,创建一个`MinMaxScaler`对象:
```python
scaler = MinMaxScaler()
```
接下来,将要归一化的数据传递给`fit_transform`方法:
```python
normalized_data = scaler.fit_transform(data)
```
这将返回归一化后的数据。请注意,`fit_transform`方法会同时拟合和转换数据。如果你已经拟合了一个`MinMaxScaler`对象,并且想将另一个数据集应用于相同的转换,你可以使用`transform`方法而不是`fit_transform`方法:
```python
normalized_data = scaler.transform(new_data)
```
这样就可以将新的数据集进行归一化处理。
相关推荐
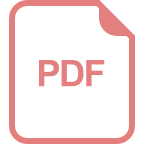
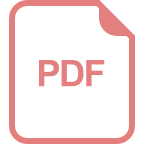












