Tiny RTC 12C 时钟模块的使用方法
时间: 2024-05-20 20:15:27 浏览: 144
Tiny RTC 12C时钟模块是一种实时时钟模块,具有高精度、低功耗、易于集成等特点,常用于电子设备中对时间进行计时和计量。下面介绍一下其使用方法:
1. 连接硬件:将Tiny RTC 12C时钟模块的SDA和SCL引脚分别连接到控制器的I2C总线上,同时连接VCC和GND引脚到电源。
2. 初始化设置:通过I2C总线向时钟模块发送指令,进行初始化设置。例如,可以设置时钟模块的时间、日期、闹钟等信息。
3. 读取时间:通过I2C总线向时钟模块发送指令,读取时钟模块当前的时间信息。可以将这些信息显示在LCD屏幕上或者通过串口输出到电脑端。
4. 定时任务:使用时钟模块的定时功能,可以实现各种定时任务。例如,可以设置每天定时开启或关闭设备,或者定时采集传感器数据等。
需要注意的是,使用Tiny RTC 12C时钟模块前需要先了解I2C总线的基本原理和使用方法。同时,在进行硬件连接和初始化设置时,需要参考时钟模块的使用手册和示例代码。
相关问题
Tiny RTC 12C 时钟模块
Tiny RTC 12C时钟模块是一种集成了实时时钟(RTC)和温度补偿水晶振荡器(TCXO)的小型电路板。它能够提供高精度的时间和日期信息,并且可以通过I2C接口与微控制器或其他电子设备进行通信。这种时钟模块广泛应用于各种电子设备中,例如数字时钟、计时器、数据记录器、监控系统等。由于其小巧、低功耗、高精度等特点,它在物联网、智能家居等领域也有广泛应用。
Tiny RTC 12C 时钟模块的初始化设置
Tiny RTC 12C时钟模块的初始化设置需要以下几个步骤:
1. 连接硬件:将Tiny RTC 12C时钟模块与单片机或开发板连接,其中SCL和SDA分别连接到单片机或开发板的I2C总线上。
2. 开启I2C总线:在单片机或开发板上开启I2C总线,使其能够与Tiny RTC 12C时钟模块进行通信。
3. 设置时钟:在初始化时,需要设置Tiny RTC 12C时钟模块的时间和日期。这可以通过写入特定的寄存器来完成。例如,可以将当前的时间和日期写入DS1307芯片的0x00-0x06寄存器中。
4. 启用时钟:在完成上述设置后,需要将Tiny RTC 12C时钟模块的时钟启用。这可以通过将控制寄存器中的特定位设置为1来完成。例如,可以将DS1307芯片的0x00寄存器中的CH位设置为0,表示启用时钟。
5. 定时更新:为了确保时钟的准确性,可以使用定时器来定期更新Tiny RTC 12C时钟模块。例如,可以设置一个每秒中断以更新时钟。
阅读全文
相关推荐
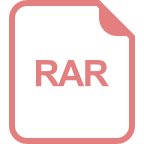
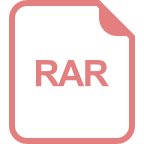
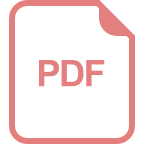

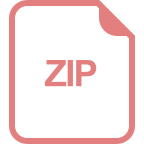
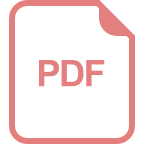
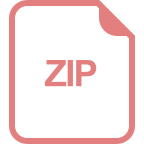
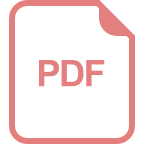
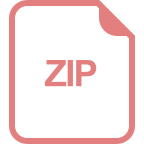
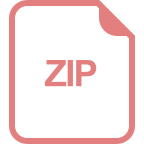
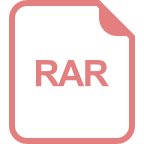
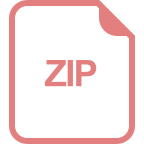
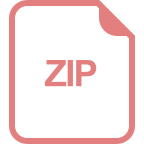
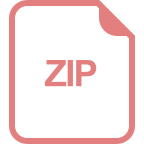
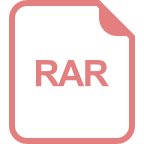
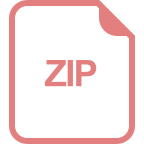