把一个1200*900像素的图片resize为28*28并展开成(1,784)的数组
时间: 2023-11-27 13:03:58 浏览: 49
可以使用Python的PIL库来对图片进行resize,然后使用numpy库将图片转换为(1, 784)的数组。
以下是实现的代码示例:
```python
from PIL import Image
import numpy as np
# 加载原始图片
img = Image.open('input_image.png')
# 将图片resize为28*28
img = img.resize((28, 28))
# 将图片转换为灰度图
img = img.convert('L')
# 将图片转换为numpy数组
img_array = np.array(img)
# 将二维数组展开为一维数组
img_array = img_array.reshape(1, -1)
# 输出展开后的数组
print(img_array)
```
在代码中,我们首先使用PIL库的`Image.open()`函数加载原始图片,然后使用`resize()`函数将图片resize为28*28像素大小。接着,我们将图片转换为灰度图,并使用`np.array()`函数将其转换为numpy数组。最后,我们使用`reshape()`函数将二维数组展开为一维数组,得到了(1, 784)的数组。
相关问题
根据输入的图片元素内容映射每个像素点到一张新的VR全景图中,类似地球展开的全景图方式,参数1: 图像元素文件路径 参数2: 图像元素内容在VR全景图的起始坐标 参数2: 输出VR全景图大小 参数3: 输出VR全景文件路径 ;使用C++实现,使用球形映射算法,不使用opengl;
实现这个功能需要以下步骤:
1. 加载图像元素文件,获取其宽度和高度。
2. 创建输出VR全景图像,大小为给定的输出VR全景图大小。
3. 使用球形映射算法,将图像元素内容映射到VR全景图中。球形映射算法的思路是将球体展开成一个平面,然后将图像元素内容映射到展开后的平面上。具体实现方式可以参考以下步骤:
a. 首先,将输出VR全景图像的中心点设置为球体的北极点(即上方)。
b. 然后,对于输出VR全景图像的每个像素点,计算该像素点在球体上的对应点的经纬度坐标。
c. 接着,将球体上的经纬度坐标转换为展开后平面上的坐标。
d. 最后,将图像元素内容映射到展开后平面上的对应位置。
4. 保存输出VR全景图像到给定的输出VR全景文件路径。
下面是一个简单的 C++ 实现代码示例:
```c++
#include <iostream>
#include <fstream>
#include <cmath>
#include <vector>
#include <algorithm>
using namespace std;
// 球面坐标
struct SphericalCoord {
double theta; // 极角
double phi; // 经角
};
// 图像元素
struct ImageElement {
int x, y; // 图像元素在 VR 全景图中的起始坐标
vector<vector<int>> data; // 图像元素数据
};
// 将度数转换为弧度
double deg2rad(double deg) {
return deg * M_PI / 180.0;
}
// 将弧度转换为度数
double rad2deg(double rad) {
return rad * 180.0 / M_PI;
}
// 计算两个球面坐标之间的距离
double dist(SphericalCoord p1, SphericalCoord p2) {
double cos_theta = sin(p1.theta) * sin(p2.theta) * cos(p1.phi - p2.phi) + cos(p1.theta) * cos(p2.theta);
return acos(cos_theta);
}
// 计算球面坐标对应的二维平面坐标
pair<int, int> spherical2planar(SphericalCoord coord, int width, int height) {
double x = (coord.phi + M_PI) * width / (2 * M_PI);
double y = (M_PI / 2 - coord.theta) * height / M_PI;
return make_pair(round(x), round(y));
}
// 计算二维平面坐标对应的球面坐标
SphericalCoord planar2spherical(pair<int, int> coord, int width, int height) {
double phi = (double)coord.first * 2 * M_PI / width - M_PI;
double theta = M_PI / 2 - (double)coord.second * M_PI / height;
return { theta, phi };
}
// 加载图像元素
ImageElement loadImageElement(string filepath, int startX, int startY) {
ImageElement element;
element.x = startX;
element.y = startY;
ifstream fin(filepath);
if (!fin) {
cerr << "Failed to open file: " << filepath << endl;
return element;
}
int width, height;
fin >> width >> height;
element.data.resize(height, vector<int>(width));
for (int y = 0; y < height; ++y) {
for (int x = 0; x < width; ++x) {
fin >> element.data[y][x];
}
}
return element;
}
// 创建空的 VR 全景图像
vector<vector<int>> createEmptyImage(int width, int height) {
return vector<vector<int>>(height, vector<int>(width));
}
// 将图像元素映射到 VR 全景图像
void mapImageElementToImage(ImageElement element, vector<vector<int>>& image) {
int startX = element.x;
int startY = element.y;
int endX = startX + element.data[0].size();
int endY = startY + element.data.size();
for (int y = startY; y < endY; ++y) {
for (int x = startX; x < endX; ++x) {
auto coord = planar2spherical(make_pair(x, y), image[0].size(), image.size());
auto newCoord = spherical2planar({ coord.first, coord.second - M_PI / 2 }, element.data[0].size(), element.data.size());
if (newCoord.first >= 0 && newCoord.first < element.data[0].size() && newCoord.second >= 0 && newCoord.second < element.data.size()) {
image[y][x] = element.data[newCoord.second][newCoord.first];
}
}
}
}
// 保存 VR 全景图像
void saveImage(string filepath, vector<vector<int>> image) {
ofstream fout(filepath);
if (!fout) {
cerr << "Failed to open file: " << filepath << endl;
return;
}
fout << image[0].size() << " " << image.size() << endl;
for (int y = 0; y < image.size(); ++y) {
for (int x = 0; x < image[0].size(); ++x) {
fout << image[y][x] << " ";
}
fout << endl;
}
}
int main() {
// 输入参数
string elementFilepath = "element.txt";
int startX = 0;
int startY = 0;
int outputWidth = 1024;
int outputHeight = 512;
string outputFilepath = "output.txt";
// 加载图像元素
auto element = loadImageElement(elementFilepath, startX, startY);
// 创建空的 VR 全景图像
auto image = createEmptyImage(outputWidth, outputHeight);
// 将图像元素映射到 VR 全景图像
mapImageElementToImage(element, image);
// 保存 VR 全景图像
saveImage(outputFilepath, image);
return 0;
}
```
其中,`loadImageElement` 函数用于加载图像元素,`createEmptyImage` 函数用于创建空的 VR 全景图像,`mapImageElementToImage` 函数用于将图像元素映射到 VR 全景图像,`saveImage` 函数用于保存 VR 全景图像。注意,这里的 VR 全景图像是以二维数组的形式存储的,每个元素表示一个像素点的颜色值。在实际应用中,可能需要将其转换为图片格式(如 JPG、PNG 等)以便于显示和使用。
import numpy as np import tensorflow as tf from tensorflow.keras.models import Sequential from tensorflow.keras.layers import Dense, Flatten from tensorflow.keras.callbacks import EarlyStopping from PIL import Image # 加载数据集 X = [] y = [] for i in range(1000): img = Image.open(f"train/cat.{i}.jpg") img = img.resize((150, 150)) X.append(np.array(img)) y.append(0) for i in range(1000): img = Image.open(f"train/dog.{i}.jpg") img = img.resize((150, 150)) X.append(np.array(img)) y.append(1) X = np.array(X) y = np.array(y) # 划分训练集和测试集 X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # 构建模型 model = Sequential([ Flatten(input_shape=(150, 150, 3)), Dense(128, activation='relu'), Dense(1, activation='sigmoid') ]) # 编译模型 model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy']) # 训练模型 early_stopping = EarlyStopping(monitor='val_loss', patience=3) model.fit(X_train, y_train, epochs=10, validation_data=(X_test, y_test), callbacks=[early_stopping]) # 计算准确率 loss, acc = model.evaluate(X_test, y_test) print(f"Accuracy: {acc}")
这段代码是一个使用卷积神经网络做猫狗分类的例子。
首先,代码通过PIL库加载了一千张猫和一千张狗的图片,并将它们缩放成150x150像素的大小。然后将图片数据存储到数组X中,将标签(0表示猫,1表示狗)存储到数组y中。
接下来,使用train_test_split函数将数据集划分为训练集和测试集。
然后,使用Sequential模型构建了一个简单的卷积神经网络模型。这个模型包含了一个Flatten层(将图片数据展开成一维向量)、一个Dense层、和一个输出层。其中Dense层使用了ReLU激活函数,输出层使用了sigmoid激活函数,用于输出分类结果(猫或狗)的概率。
模型中使用了Adam优化器和二元交叉熵损失函数,并用accuracy评估了模型的性能。
在训练模型时,使用了EarlyStopping回调函数,以防止过拟合。
最后,代码计算并打印了模型在测试集上的准确率。
阅读全文
相关推荐
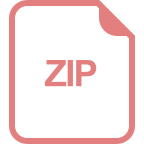
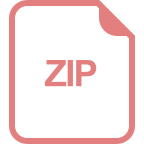
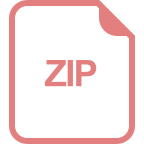
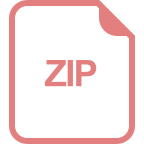
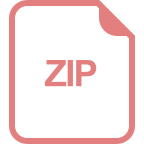
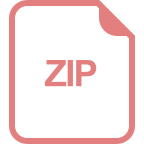
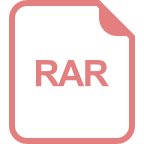
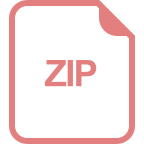