点击Dropdown组件的menu打开弹窗后,menu不消失怎么处理
时间: 2024-06-05 22:10:16 浏览: 7
通常情况下,Dropdown组件的menu打开弹窗后,点击弹窗以外的区域或者ESC键都会使得menu消失。如果menu不消失,可能是以下几个原因导致:
1. 弹窗未正常关闭:可能是因为弹窗未正常关闭,导致menu无法消失。你可以检查一下弹窗的关闭逻辑是否正确。
2. 事件捕获的问题:可能是由于事件捕获导致的。你可以在打开弹窗的时候,将事件捕获到弹窗组件上。这样点击弹窗以外的区域时,事件就会被捕获到弹窗组件上,弹窗就会正常关闭,menu也就能够消失了。
3. CSS的问题:可能是由于CSS的问题导致的。你可以检查一下CSS样式是否正确,特别是对于menu的定位和z-index属性的设置是否正确。
如果以上方法都不能解决问题,可以尝试在相关的论坛或者社区发帖求助,或者咨询相关的技术支持人员。
相关问题
鼠标移入Dropdown组件的menu,导致原组件的hover消失怎么解决
这个问题可能是由于CSS样式覆盖或者事件冒泡导致的。下面是一些可能的解决方案:
1. 使用CSS优先级来覆盖Dropdown组件的hover样式。例如,可以给Dropdown组件添加一个更高优先级的CSS类来保留hover样式。
2. 在Dropdown组件的menu中添加一个事件监听器,阻止事件冒泡到Dropdown组件,从而防止hover效果被覆盖。例如,在menu的鼠标移入事件处理程序中添加以下代码:
```
event.stopPropagation();
```
3. 调整Dropdown组件的HTML结构,使menu与原组件hover效果不会相互影响。例如,可以将menu移动到原组件的外部,并使用CSS定位来实现下拉菜单的效果。
以上是一些可能的解决方案,具体取决于你的代码实现和具体情况,希望能够对你有所帮助。
van-dropdown-menu组件使用介绍
Van-dropdown-menu是Vanilla-UI库中的一个下拉菜单组件,用于在页面中展示一个菜单。使用该组件需要先引入Vanilla-UI库,并在需要使用的页面中进行初始化配置。
该组件的使用方法如下:
1. 引入Vanilla-UI库
2. 在HTML文件中定义下拉菜单的触发元素和菜单元素,设置它们的class名为van-dropdown-menu和van-dropdown-menu__list
3. 初始化VanDropdownMenu对象并进行相应的配置,如:设置默认选项、设置菜单最大高度、设置菜单显示方向等。
以下是一个示例:
```
<!-- 引入Vanilla-UI库 -->
<link rel="stylesheet" href="path/to/vanilla-ui.css">
<script src="path/to/vanilla-ui.js"></script>
<!-- 定义触发元素和菜单元素 -->
<button class="van-dropdown-menu">展示菜单</button>
<ul class="van-dropdown-menu__list" style="display:none;">
<li>菜单项1</li>
<li>菜单项2</li>
<li>菜单项3</li>
</ul>
<script>
// 初始化VanDropdownMenu对象并进行相应的配置
new VanDropdownMenu('.van-dropdown-menu', {
defaultIndex: 0,
maxHeight: 200,
direction: 'bottom'
});
</script>
```
通过调用VanDropdownMenu对象的方法,可以实现菜单的打开、关闭、选项的切换等操作。
相关推荐
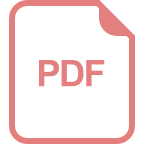
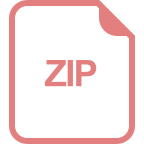
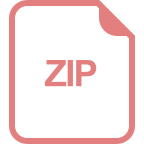












