1、 实现一个Winform程序,要求实现如下功能: 1)程序能够记忆位置 2)程序能够记忆大小 3)该界面中的内容能够自动保存(命名为 d:\yyyy-mm-dd hh-mm-ss¬-4位随机数.txt),保存间隔时间自定
时间: 2024-02-05 21:10:51 浏览: 120
以下是一个实现上述功能的Winform程序的示例代码:
```c#
using System;
using System.IO;
using System.Windows.Forms;
namespace WinformMemo
{
public partial class MainForm : Form
{
private const string saveFolder = @"d:\";
private const int saveInterval = 60; // 保存间隔时间,单位为秒
private string savedFilePath;
private DateTime lastSaveTime;
public MainForm()
{
InitializeComponent();
// 读取上一次保存的位置和大小
if (Properties.Settings.Default.WindowLocation != null)
{
this.StartPosition = FormStartPosition.Manual;
this.Location = Properties.Settings.Default.WindowLocation;
}
if (Properties.Settings.Default.WindowSize != null)
{
this.Size = Properties.Settings.Default.WindowSize;
}
// 开始自动保存计时器
this.lastSaveTime = DateTime.Now;
this.autoSaveTimer.Interval = saveInterval * 1000;
this.autoSaveTimer.Start();
}
private void MainForm_FormClosing(object sender, FormClosingEventArgs e)
{
// 保存当前位置和大小
Properties.Settings.Default.WindowLocation = this.Location;
Properties.Settings.Default.WindowSize = this.Size;
Properties.Settings.Default.Save();
// 手动保存一次
SaveData();
}
private void autoSaveTimer_Tick(object sender, EventArgs e)
{
// 每隔一段时间自动保存一次
if ((DateTime.Now - this.lastSaveTime).TotalSeconds >= saveInterval)
{
SaveData();
}
}
private void SaveData()
{
// 生成保存文件路径
string fileName = $"{DateTime.Now.ToString("yyyy-MM-dd HH-mm-ss")}-{new Random().Next(1000, 9999)}.txt";
this.savedFilePath = Path.Combine(saveFolder, fileName);
// 保存界面中的内容
using (StreamWriter writer = new StreamWriter(this.savedFilePath))
{
writer.Write(this.textBox.Text);
}
// 更新最后保存时间
this.lastSaveTime = DateTime.Now;
}
}
}
```
该程序使用了Windows Forms框架,具有以下功能:
- 记忆窗口位置和大小,下次打开程序时自动还原;
- 每隔一段时间自动保存界面中的文本内容,并命名为当前时间和4位随机数组成的文件名,保存在指定的文件夹中。
程序界面中只有一个多行文本框(textBox),通过在窗体的Closing事件中保存窗口位置和大小,然后在窗体加载时读取上一次保存的位置和大小来实现位置和大小的记忆。
自动保存功能通过一个计时器(autoSaveTimer)来实现。该计时器在窗体加载时开始运行,每隔一定的时间(即保存间隔时间,单位为秒)触发一次Tick事件,在该事件中执行保存操作。同时,也在窗体的Closing事件中手动保存一次,以确保最后一次编辑的内容能被保存。
注意,为了让程序能够记忆窗口位置和大小,需要在窗体设计器中将StartPosition属性设置为Manual。另外,为了让程序在下次打开时能够自动还原位置和大小,需要在窗体的Shown事件中调用this.Activate()方法,以确保当前窗口是激活状态。
阅读全文
相关推荐
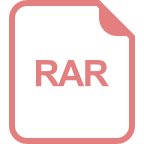
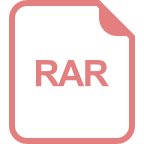
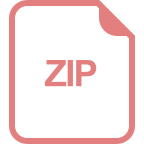
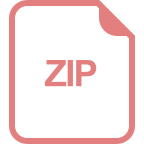
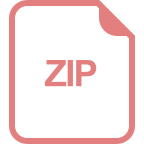
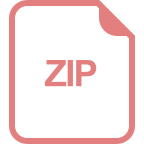
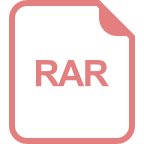
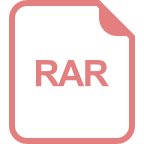
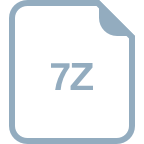
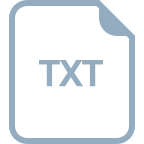
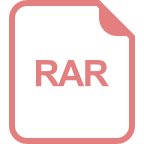
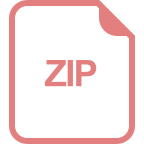
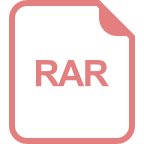
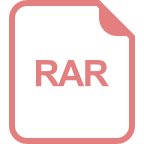
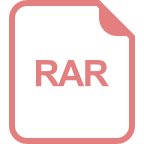
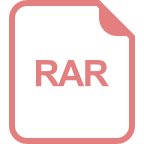
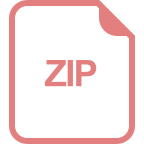
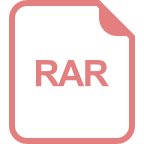