java多线程断点续传下载
时间: 2023-11-01 13:55:29 浏览: 44
实现多线程断点续传下载需要以下步骤:
1. 获取文件大小和已下载的大小,计算出剩余未下载的文件大小。
2. 开启多个线程,每个线程负责下载文件的一部分内容。每个线程要知道自己需要下载的文件范围,即从哪里开始下载,下载多少字节。
3. 每个线程要在自己的范围内不断地读取数据,并写入文件。同时要记录已经下载的字节数。
4. 如果下载过程中出现错误,可以将已下载的字节数保存到本地文件中,下次启动程序时读取该文件,接着下载。
5. 下载完成后,合并所有下载的数据,得到完整的文件。
下面是一个示例代码:
```
import java.io.*;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.ArrayList;
import java.util.List;
public class MultiThreadDownloader {
private static final String DOWNLOAD_DIR = "C:/download/";
private static final int THREAD_COUNT = 4;
public static void main(String[] args) {
String url = "http://example.com/file.zip";
String fileName = url.substring(url.lastIndexOf("/") + 1);
try {
URL fileUrl = new URL(url);
HttpURLConnection conn = (HttpURLConnection) fileUrl.openConnection();
int fileSize = conn.getContentLength();
System.out.println("File size: " + fileSize);
List<DownloadThread> threads = new ArrayList<>();
int blockSize = fileSize / THREAD_COUNT;
for (int i = 0; i < THREAD_COUNT; i++) {
int start = i * blockSize;
int end = (i + 1) * blockSize - 1;
if (i == THREAD_COUNT - 1) {
end = fileSize - 1;
}
DownloadThread t = new DownloadThread(url, DOWNLOAD_DIR + fileName, start, end);
t.start();
threads.add(t);
}
for (DownloadThread t : threads) {
t.join();
}
mergeFile(DOWNLOAD_DIR + fileName, THREAD_COUNT);
System.out.println("Download complete");
} catch (Exception e) {
e.printStackTrace();
}
}
private static void mergeFile(String fileName, int threadCount) throws IOException {
BufferedOutputStream out = new BufferedOutputStream(new FileOutputStream(fileName));
for (int i = 0; i < threadCount; i++) {
BufferedInputStream in = new BufferedInputStream(new FileInputStream(fileName + "." + i));
byte[] buffer = new byte[1024];
int len;
while ((len = in.read(buffer)) != -1) {
out.write(buffer, 0, len);
}
in.close();
File file = new File(fileName + "." + i);
file.delete();
}
out.close();
}
private static class DownloadThread extends Thread {
private String url;
private String fileName;
private int start;
private int end;
public DownloadThread(String url, String fileName, int start, int end) {
this.url = url;
this.fileName = fileName;
this.start = start;
this.end = end;
}
@Override
public void run() {
try {
File file = new File(fileName + "." + getId());
RandomAccessFile raf = new RandomAccessFile(file, "rw");
HttpURLConnection conn = (HttpURLConnection) new URL(url).openConnection();
conn.setRequestProperty("Range", "bytes=" + start + "-" + end);
BufferedInputStream in = new BufferedInputStream(conn.getInputStream());
byte[] buffer = new byte[1024];
int len, progress = 0;
while ((len = in.read(buffer)) != -1) {
raf.write(buffer, 0, len);
progress += len;
}
raf.close();
in.close();
System.out.println("Thread " + getId() + " download complete (" + progress + " bytes)");
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
```
在这个示例代码中,我们使用了4个线程来下载文件。每个线程下载的范围计算方式为:第i个线程下载的范围是[start, end],其中start=i*blockSize,end=(i+1)*blockSize-1。最后一个线程的end是文件的总大小减1。每个线程下载完后,将下载的数据保存到本地文件中,文件名为原文件名加上线程ID。下载完成后,我们将所有下载的文件合并成一个完整的文件。
相关推荐
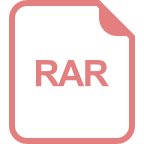
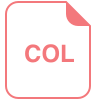
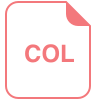
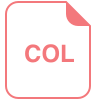
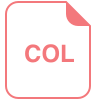
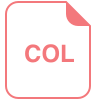
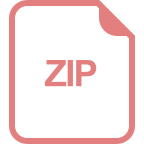
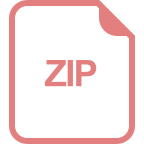
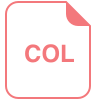
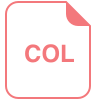
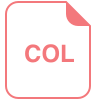
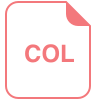
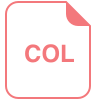
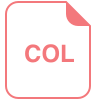
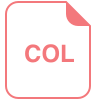