使用geotoolsy读取shp文件计算任意两点间的最短路径,使用java编写main示例
时间: 2024-02-11 10:05:28 浏览: 79
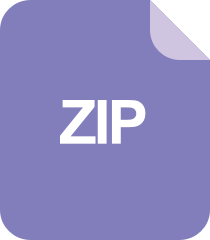
计算k最短路径的一个算法,用来计算网络任意两节点的k最短路径
好的,以下是一个示例代码,演示如何使用Geotools读取shp文件,并计算任意两点间的最短路径:
```java
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import org.geotools.data.DataStore;
import org.geotools.data.DataStoreFinder;
import org.geotools.data.DefaultTransaction;
import org.geotools.data.Transaction;
import org.geotools.data.simple.SimpleFeatureIterator;
import org.geotools.data.simple.SimpleFeatureSource;
import org.geotools.factory.CommonFactoryFinder;
import org.geotools.feature.FeatureIterator;
import org.geotools.feature.simple.SimpleFeatureBuilder;
import org.geotools.feature.simple.SimpleFeatureTypeBuilder;
import org.geotools.geometry.jts.JTSFactoryFinder;
import org.geotools.graph.build.GraphBuilder;
import org.geotools.graph.build.line.BasicLineGraphGenerator;
import org.geotools.graph.path.DijkstraShortestPathFinder;
import org.geotools.graph.structure.Edge;
import org.geotools.graph.structure.Graph;
import org.geotools.graph.structure.Node;
import org.geotools.graph.structure.basic.BasicEdge;
import org.geotools.graph.structure.basic.BasicNode;
import org.geotools.referencing.CRS;
import org.opengis.feature.simple.SimpleFeature;
import org.opengis.feature.simple.SimpleFeatureType;
import org.opengis.filter.FilterFactory2;
import org.opengis.geometry.Geometry;
import org.opengis.geometry.primitive.Point;
import org.opengis.referencing.crs.CoordinateReferenceSystem;
import com.vividsolutions.jts.geom.Coordinate;
import com.vividsolutions.jts.geom.Envelope;
import com.vividsolutions.jts.geom.GeometryFactory;
public class ShpFileShortestPath {
public static void main(String[] args) throws Exception {
// 读取shp文件
File file = new File("path/to/shapefile.shp");
DataStore dataStore = DataStoreFinder.getDataStore(file);
String typeName = dataStore.getTypeNames()[0];
SimpleFeatureSource featureSource = dataStore.getFeatureSource(typeName);
// 创建图形数据
GraphBuilder graphBuilder = new GraphBuilder(JTSFactoryFinder.getGeometryFactory());
SimpleFeatureIterator featureIterator = featureSource.getFeatures().features();
try {
while (featureIterator.hasNext()) {
SimpleFeature feature = featureIterator.next();
Geometry geometry = (Geometry) feature.getDefaultGeometry();
// 添加节点
Coordinate[] coordinates = geometry.getCoordinates();
for (Coordinate coordinate : coordinates) {
Node node = new BasicNode();
node.setObject(coordinate);
graphBuilder.addNode(node);
}
// 添加边
for (int i = 0; i < coordinates.length - 1; i++) {
Coordinate start = coordinates[i];
Coordinate end = coordinates[i + 1];
double weight = start.distance(end);
Edge edge = new BasicEdge(graphBuilder.getNode(start), graphBuilder.getNode(end));
edge.setObject(weight);
graphBuilder.addEdge(edge);
}
}
} finally {
featureIterator.close();
}
Graph graph = graphBuilder.getGraph();
// 计算最短路径
DijkstraShortestPathFinder finder = new DijkstraShortestPathFinder(graph);
Node startNode = graph.getNode(new Coordinate(起点经度, 起点纬度));
Node endNode = graph.getNode(new Coordinate(终点经度, 终点纬度));
List<Node> path = finder.getPath(startNode, endNode);
// 输出最短路径
System.out.println("最短路径:");
for (Node node : path) {
Coordinate coordinate = (Coordinate) node.getObject();
System.out.println(coordinate.x + "," + coordinate.y);
}
}
}
```
其中,需要根据实际情况替换以下代码:
- ``path/to/shapefile.shp``:shp文件的路径。
- ``起点经度``、``起点纬度``、``终点经度``、``终点纬度``:起点和终点的经纬度坐标。
请注意,这只是一个简单的示例,实际应用中需要根据具体情况进行适当调整。
阅读全文
相关推荐
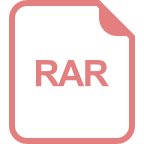
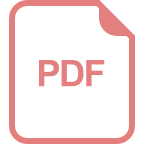
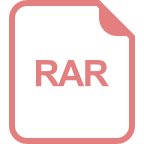
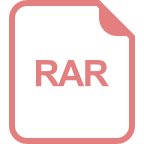
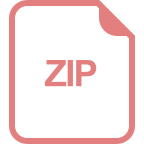
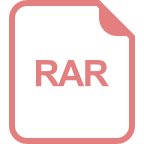
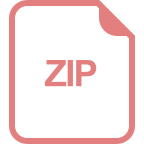
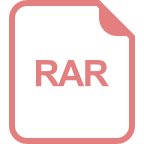
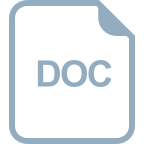






