protected void gvInfo_RowDataBound(object sender, GridViewRowEventArgs e) { //鼠标移动变色 if (e.Row.RowType == DataControlRowType.DataRow) { //当鼠标放上去的时候 先保存当前行的背景颜色 并给附一颜色 e.Row.Attributes.Add("onmouseover", "currentcolor=this.style.backgroundColor;this.style.backgroundColor='#f6f6f6',this.style.fontWeight='';"); //当鼠标离开的时候 将背景颜色还原的以前的颜色 e.Row.Attributes.Add("onmouseout", "this.style.backgroundColor=currentcolor,this.style.fontWeight='';"); } //单击行改变行背景颜色 if (e.Row.RowType == DataControlRowType.DataRow) { e.Row.Attributes.Add("onclick", "this.style.backgroundColor='#f6f6f6'; this.style.color='buttontext';this.style.cursor='default';"); } } protected void gvOrderInfo_PageIndexChanging(object sender, GridViewPageEventArgs e) { gvOrderInfo.PageIndex = e.NewPageIndex; Get_Info(); }
时间: 2024-04-06 17:28:05 浏览: 145
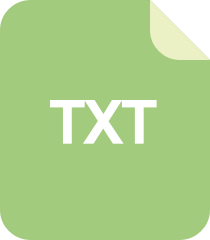
嵌套的在GridView里的radiobutton 怎么才能只选中一个_asp_net(c#)

这是一个 C# 代码片段,包含两个方法。第一个方法名为 "gvInfo_RowDataBound",在 GridView 绑定数据的时候会被调用,作用是为 GridView 的每一行添加特定的鼠标事件,实现鼠标悬停和离开时的背景颜色变化和文字加粗功能。第二个方法名为 "gvOrderInfo_PageIndexChanging",是 GridView 分页时的事件处理程序,用于处理分页控件的换页事件,将当前页码设置为新页码,并重新绑定数据源。
阅读全文
相关推荐
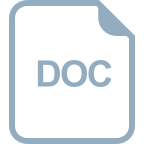
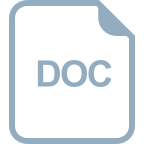
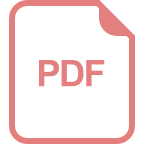
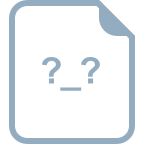
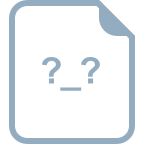
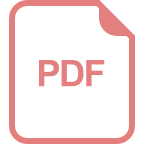
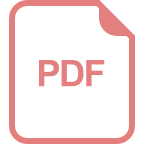
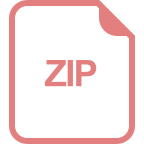
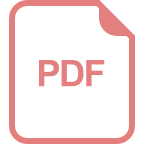
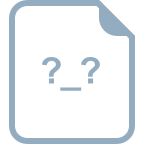
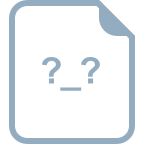
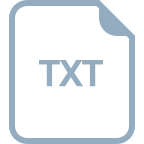
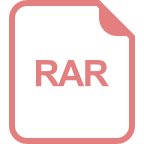
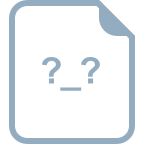
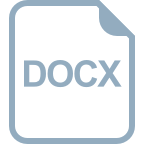


