jframe单选框判断是否勾选
时间: 2023-09-28 22:13:50 浏览: 131
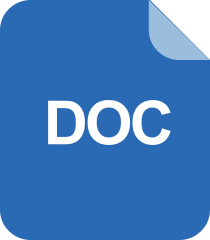
jQuery如何判断checkbox(复选框)是否被选中
在Java Swing中,可以通过JRadioButton和ButtonGroup实现单选框的选择。若要判断单选框是否被选中,可以使用isSelected()方法来判断。
具体实现方法如下:
1.首先创建一个JRadioButton对象,例如:
JRadioButton radioButton = new JRadioButton("单选框");
2.创建一个ButtonGroup对象,并将JRadioButton对象添加到ButtonGroup中,例如:
ButtonGroup buttonGroup = new ButtonGroup();
buttonGroup.add(radioButton);
3.判断单选框是否被选中,例如:
if (radioButton.isSelected()) {
System.out.println("单选框被选中");
} else {
System.out.println("单选框未被选中");
}
以上代码片段可以在Java Swing应用程序的ActionListener事件中使用,以便在用户单击按钮时执行相应的操作。
阅读全文
相关推荐
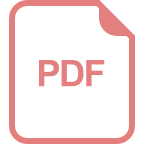
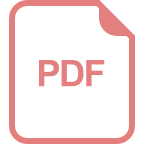

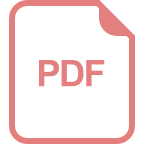
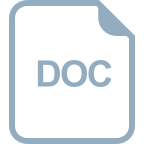




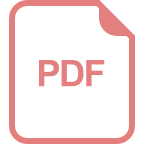
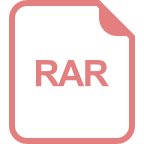
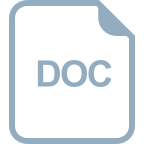
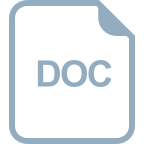
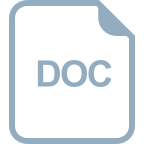
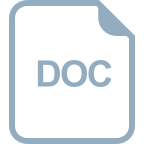
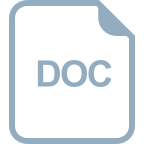

