基于C++,设计一个数组类模板,类外部可以通过下标初始化数组元素,类中有求和函数
时间: 2023-06-02 14:06:16 浏览: 178
抱歉,我能够回答这个问题。以下是基于C++设计的数组类模板,包含了初始化数组元素和求和函数的实现:
```c++
template<class T, int size>
class Array {
private:
T arr[size];
public:
Array() {
for (int i = 0; i < size; i++)
arr[i] = T(); // initialize each element with default value
}
T& operator[](int index) {
if (index >= size || index < 0) throw "Index out of bounds";
return arr[index];
}
T sum() {
T sum = T();
for (int i = 0; i < size; i++)
sum += arr[i];
return sum;
}
};
```
使用示例:
```c++
Array<int, 5> nums;
nums[0] = 1;
nums[1] = 2;
nums[2] = 3;
nums[3] = 4;
nums[4] = 5;
cout << nums.sum() << endl; // Output: 15
```
相关问题
c++用模板类设计一个数组类模板
好的,下面是一个简单的用模板类设计的数组类模板:
```c++
template <typename T, int size>
class Array {
private:
T data[size];
public:
Array() {} // 默认构造函数
// 拷贝构造函数
Array(const Array<T, size>& other) {
for (int i = 0; i < size; i++) {
data[i] = other.data[i];
}
}
// 重载数组操作符[]
T& operator[](int index) {
return data[index];
}
// 返回数组大小
int getSize() const {
return size;
}
};
```
这个数组类模板接受两个参数:数据类型 `T` 和数组大小 `size`。它包含默认构造函数、拷贝构造函数、重载数组操作符 `[]` 和返回数组大小的函数 `getSize()`。可以通过以下方式实例化一个 `Array` 对象:
```c++
Array<int, 5> myArray; // 创建一个 int 类型的长度为 5 的数组
myArray[0] = 1; // 访问数组元素
int size = myArray.getSize(); // 返回数组大小
```
希望这个例子可以帮助你理解用模板类设计数组类模板的基本思路。
c++设计一个类模板 Array,用来实现可以任意指定下标范围的任意类型的 数组。
好的,那么我为您设计一个类模板 Array,它可以实现任意指定下标范围的任意类型的数组,以下是示例代码:
```cpp
#include <iostream>
#include <stdexcept>
template <typename T, int start_index, int end_index>
class Array {
public:
Array() {
for (int i = start_index; i <= end_index; ++i) {
data_[i - start_index] = T{};
}
}
T& operator[](int index) {
if (index < start_index || index > end_index) {
throw std::out_of_range("Index out of range");
}
return data_[index - start_index];
}
void print() const {
std::cout << "[ ";
for (int i = start_index; i <= end_index; ++i) {
std::cout << data_[i - start_index] << " ";
}
std::cout << "]" << std::endl;
}
private:
T data_[end_index - start_index + 1];
};
```
这个类模板以 T 作为存储数据的类型,以 start_index 作为数组的起始下标,以 end_index 作为数组的结束下标。它重载了 [] 运算符,使得可以使用下标访问数组元素,同时进行边界检查。如果下标越界,则抛出 std::out_of_range 异常。
使用方法如下:
```cpp
int main() {
Array<int, -2, 2> arr;
arr.print();
// 输出:[ 0 0 0 0 0 ]
arr[0] = 1;
arr[-2] = 2;
arr[2] = 3;
arr.print();
// 输出:[ 2 0 1 0 3 ]
return 0;
}
```
在这个示例中,我们创建了一个起始下标为 -2,结束下标为 2 的整型数组,并对其进行了赋值和输出。输出结果为:
```
[ 0 0 0 0 0 ]
[ 2 0 1 0 3 ]
```
希望能对你有所帮助!
阅读全文
相关推荐

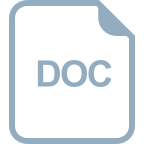














