用QT写一个查看图片的程序,要求点击“上一张”和“下一张”实现显示图片,基类为qdialog
时间: 2024-04-29 11:25:51 浏览: 14
以下是一个简单的实现:
```
#include <QDialog>
#include <QLabel>
#include <QPushButton>
#include <QHBoxLayout>
#include <QVBoxLayout>
#include <QDir>
#include <QDebug>
class ImageViewer : public QDialog
{
Q_OBJECT
public:
ImageViewer(QWidget *parent = nullptr);
private slots:
void onPreviousClicked();
void onNextClicked();
private:
QLabel *m_imageLabel;
QPushButton *m_previousButton;
QPushButton *m_nextButton;
QStringList m_imageList;
int m_currentIndex;
};
ImageViewer::ImageViewer(QWidget *parent)
: QDialog(parent)
{
m_imageLabel = new QLabel(this);
m_previousButton = new QPushButton(tr("上一张"), this);
m_nextButton = new QPushButton(tr("下一张"), this);
QHBoxLayout *buttonLayout = new QHBoxLayout;
buttonLayout->addWidget(m_previousButton);
buttonLayout->addWidget(m_nextButton);
QVBoxLayout *mainLayout = new QVBoxLayout;
mainLayout->addWidget(m_imageLabel);
mainLayout->addLayout(buttonLayout);
setLayout(mainLayout);
// 查找图片文件
QDir imagesDir(QCoreApplication::applicationDirPath() + "/images");
QStringList filters;
filters << "*.jpg" << "*.jpeg" << "*.png" << "*.bmp";
m_imageList = imagesDir.entryList(filters, QDir::Files | QDir::NoSymLinks);
m_currentIndex = 0;
if (m_imageList.size() > 0) {
QString imagePath = imagesDir.absoluteFilePath(m_imageList.at(m_currentIndex));
m_imageLabel->setPixmap(QPixmap(imagePath));
m_imageLabel->setScaledContents(true);
}
connect(m_previousButton, &QPushButton::clicked, this, &ImageViewer::onPreviousClicked);
connect(m_nextButton, &QPushButton::clicked, this, &ImageViewer::onNextClicked);
}
void ImageViewer::onPreviousClicked()
{
if (m_imageList.size() > 0) {
m_currentIndex--;
if (m_currentIndex < 0) {
m_currentIndex = m_imageList.size() - 1;
}
QString imagePath = QDir::currentPath() + "/images/" + m_imageList.at(m_currentIndex);
m_imageLabel->setPixmap(QPixmap(imagePath));
}
}
void ImageViewer::onNextClicked()
{
if (m_imageList.size() > 0) {
m_currentIndex++;
if (m_currentIndex >= m_imageList.size()) {
m_currentIndex = 0;
}
QString imagePath = QDir::currentPath() + "/images/" + m_imageList.at(m_currentIndex);
m_imageLabel->setPixmap(QPixmap(imagePath));
}
}
```
在构造函数中,我们首先查找指定目录下所有符合条件的图片文件,然后将第一张图片显示在标签上。之后,我们连接“上一张”和“下一张”按钮的点击事件到对应的槽函数。在槽函数中,我们更新当前图片的索引值,然后根据索引值找到对应的图片文件,并将其显示在标签上。需要注意的是,当索引值越界时,我们需要将其重置到合法范围内。
相关推荐
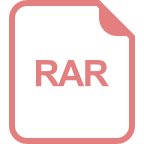
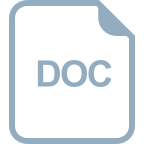
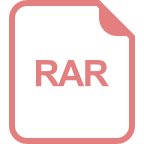














