用C++语言编写程序:从键盘输入一个字符串(注意:字符串中含有空格),使用字符数组型和字符型指针来实现程序的功能,统计且输出字符串中字母的个数。
时间: 2024-02-06 08:09:41 浏览: 72
以下是使用字符数组型实现的程序:
```c++
#include <iostream>
#include <cstring>
using namespace std;
int main()
{
const int MAX_LEN = 100;
char str[MAX_LEN];
cin.getline(str, MAX_LEN);
int count = 0;
for (int i = 0; i < strlen(str); i++) {
if (isalpha(str[i])) {
count++;
}
}
cout << "字母个数:" << count << endl;
return 0;
}
```
以下是使用字符型指针实现的程序:
```c++
#include <iostream>
#include <cstring>
using namespace std;
int main()
{
const int MAX_LEN = 100;
char* str = new char[MAX_LEN];
cin.getline(str, MAX_LEN);
int count = 0;
for (int i = 0; i < strlen(str); i++) {
if (isalpha(str[i])) {
count++;
}
}
cout << "字母个数:" << count << endl;
delete[] str;
return 0;
}
```
这两个程序的实现思路基本一致,只是一个使用了字符数组型,一个使用了字符型指针。
阅读全文
相关推荐
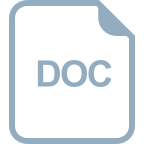
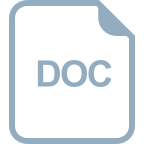
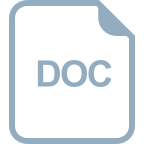

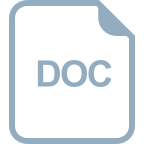
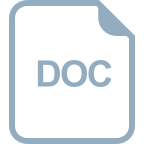
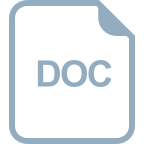
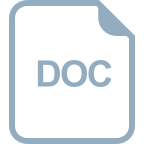
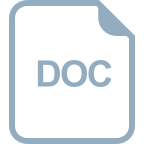
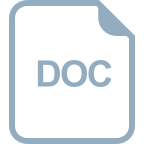
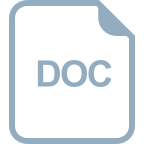
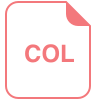



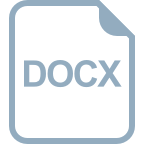