如果我想输出指定字符的下一行呢
时间: 2024-10-11 11:04:23 浏览: 21
如果你想输出文件中特定字符(比如逗号、换行符等)后的下一行,你需要先定位到该字符的位置,然后再开始读取下一行。这通常涉及到文件流的指针操作,不过在C++中,如果你的目标是在字符串内查找特定字符,并获取其之后的内容,你可能会创建一个临时缓冲区来保存这部分内容。
下面是一个基本示例,假设我们想要在每行的逗号后面读取直到换行符:
```cpp
#include <fstream>
#include <string>
std::string getNextLineAfterComma(const std::string& filename) {
std::ifstream file(filename);
if (!file.is_open()) {
throw std::runtime_error("Failed to open the file.");
}
std::string line;
std::getline(file, line);
size_t commaPos = line.find(",");
if (commaPos != std::string::npos) {
line = line.substr(commaPos + 1); // Remove everything before the comma
std::getline(file, line); // Get the next line
}
return line;
}
int main() {
try {
std::string nextLine = getNextLineAfterComma("your_file.txt");
std::cout << "The line after comma: " << nextLine << std::endl;
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
```
这里假设逗号后有内容,如果找到逗号则截取剩余部分并读取下一行。如果没有找到,`find`会返回`std::string::npos`,此时不会读取下一行。
阅读全文
相关推荐
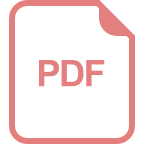
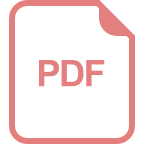
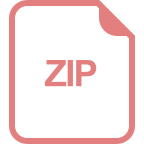
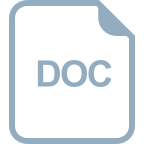
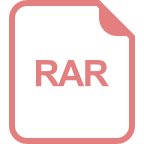
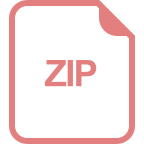
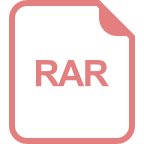
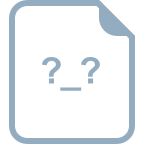
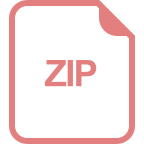
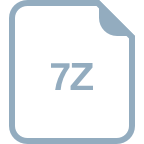
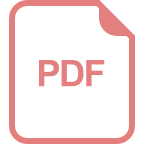
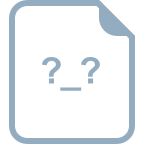
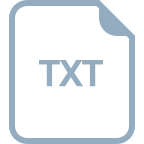
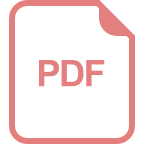
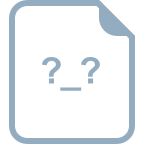
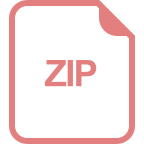
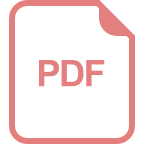
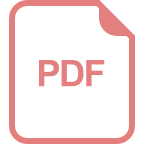
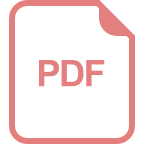