) Please write a recursive method that sums all the numbers in a given integer and returns the summation. Only recursive solutions will be marked. [12%] // Input : 178 // Output : 1*7*8 = 56 public static int multiplication(int number){ //Fill in the method body. }
时间: 2023-07-16 14:14:50 浏览: 54
Here's a recursive method in Java that sums all the digits of a given integer:
```java
public static int sumDigits(int number) {
if (number == 0) {
return 0;
} else {
return (number % 10) + sumDigits(number / 10);
}
}
```
Here's how it works:
1. The method takes an integer `number` as input.
2. If `number` is zero, the method returns zero (this is the base case).
3. Otherwise, the method returns the sum of the last digit of `number` (which is `number % 10`) and the result of calling itself recursively with the remaining digits (which is `sumDigits(number / 10)`).
For example, if we call `sumDigits(178)`, the method will compute `8 + sumDigits(17)`, which in turn will compute `7 + sumDigits(1)`, which finally returns `1`. So the overall result is `8 + 7 + 1 = 16`.
Note that this method doesn't use multiplication as in the example in the prompt. Instead, it sums the digits of the input number recursively.
相关推荐
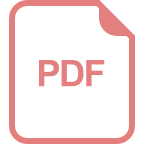
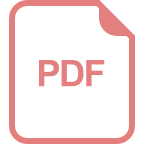














